In this PHP tutorial, you shall learn how to check if the given string starts with specific character using strpos() function, with example programs.
PHP – Check if String starts with a Specific Character
To check if string starts with a specific character, use PHP built-in function strpos(). Provide the string and character as arguments to strpos(), and if strpos() returns 0, then we can confirm that the string starts with the specific character, else not.
The syntax of condition that checks if given string starts with specific character is
strpos($string, $character) === 0
You can use this condition in a PHP If statement, and have conditional execution when a string starts with a specific character or not.
Examples
1. Check if string starts with character “H”
In this example, we will take a string, say "Hello World"
in $string, and check if this string starts with $character : "H"
. We will use an If statement with the condition specified in the introduction above.
PHP Program
<?php $string = "Hello World"; $character = "H"; if (strpos($string, $character) === 0) { echo "\"{$string}\" starts with specified character \"{$character}\"."; } else { echo "\"{$string}\" does not start with specified character \"{$character}\"."; } ?>
Output
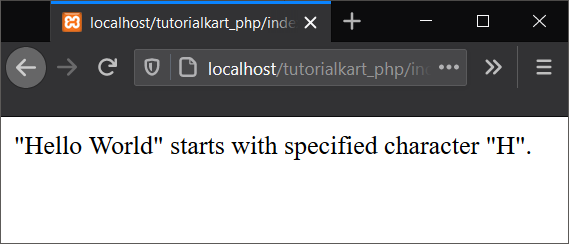
Conclusion
In this PHP Tutorial, we learned how to check if a string starts with a specific character, using PHP built-in function strpos().