In this tutorial, you shall learn about datatypes in PHP, different primitive datatypes available in PHP, with example programs.
PHP Datatypes
Datatype defines the type of value stored in a memory location, amount of space occupied by the value, and the type of operations that can done on that value.
For example, value of type integer takes four bytes, can be in the range [-2147483648, 2147483647], and two integer values can be added. Similarly a string value takes memory depending on the number of characters in it, and two string values can be concatenated.
In PHP, following datatypes are available.
- String
- Integer
- Floating Point Number (Float)
- Boolean
- Array
- Object
- NULL
The following table specifies the datatype, and example value.
Datatype | Example | Description |
---|---|---|
String | “hello world” | String is a sequence of characters. |
Integer | 145 | Integer is a non-decimal number which can be in the range [-2147483648, 2147483647] |
Float | 3.14 | Floating point number is a decimal point number or a number in exponential form. |
Boolean | true | A value of type boolean can have either of the two values: true, false. |
Array | array(‘apple’, ‘banana’, ‘cherry’) | An array can store multiple values under a single name, where each value can be accessed using index. |
Object | new Company(‘Apple’, ‘California’) | An object created from a user defined type (class). |
NULL | NULL | A NULL value can have only one value, which is NULL. NULL means nothing or no value present. |
Example
In the following example, we take values of different datatypes and print them.
PHP Program
<!DOCTYPE html> <?php //string $x = "hello world"; echo $x . "<br>"; //integer $x = 58; echo $x . "<br>"; //float $x = 3.14; echo $x . "<br>"; //boolean $x = true; echo $x . "<br>"; //array $x = array('apple', 'banana', 'cherry'); echo $x . "<br>"; //NULL $x = NULL; echo $x . "<br>"; ?>
Output
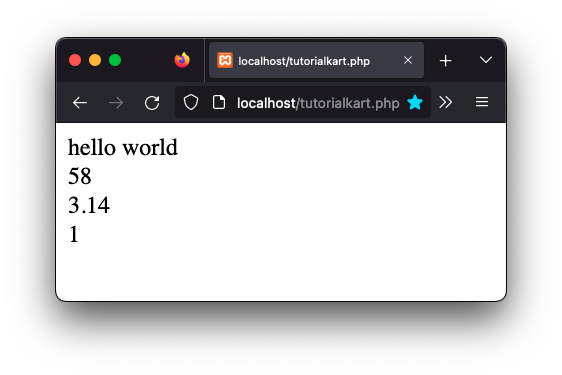
Conclusion
In this PHP Tutorial, we learned about different operators in PHP, examples for each of them. We will learn about each of them in detail in our upcoming tutorials.