Uncaught Error: Cannot access protected property
This error occurs when the property in a class is defined as protected, but accessed outside the class.
For example take the following program, where we have a class Person
with protected property $fullname
. protected means the visibility of this property is only within the class. If we try to access this property outside the class, then this fatal error is thrown.
PHP Program
<?php
class Person {
protected string $fullname;
protected int $age;
public function __construct(string $fullname = null, int $age = null) {
$this->fullname = $fullname;
$this->age = $age;
}
public function printDetails() {
echo "Name : " . $this->fullname . "<br>Age : " . $this->age;
}
}
//create object of type Person with arguments passed for constructor
$person1 = new Person("Mike Turner", 24);
//try to access protected property of class
echo $person1->fullname;
?>
Output – xampp/logs/php_error_log
[28-Feb-2023 15:33:53 Europe/Berlin] PHP Fatal error: Uncaught Error: Cannot access protected property Person::$fullname in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php:20
Stack trace:
#0 {main}
thrown in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php on line 20
Solution
If we would like to get the value of a property, we can define a getter method in the class to return the value of this property, or else define the property as public.
Solution 1 – Define a getter method to access the property
We shall define a getter method getFullname()
in the Person
class that returns the value of the property $fullname
.
PHP Program
<?php
class Person {
protected string $fullname;
protected int $age;
public function __construct(string $fullname = null, int $age = null) {
$this->fullname = $fullname;
$this->age = $age;
}
public function getFullname() {
return $this->fullname;
}
public function printDetails() {
echo "Name : " . $this->fullname . "<br>Age : " . $this->age;
}
}
//create object of type Person with arguments passed for constructor
$person1 = new Person("Abc Defg", 24);
//try to access protected property of class
echo $person1->getFullname();
?>
Output
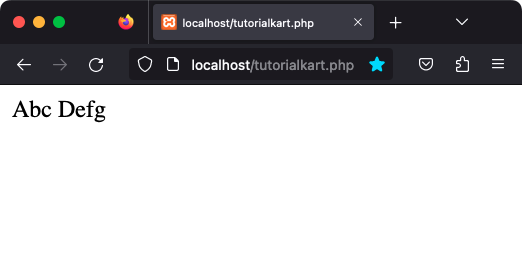
Solution 2 – Make the property public
If we can make the property $fullname
as public, then we can access the property outside the class as well.
PHP Program
<?php
class Person {
public string $fullname;
protected int $age;
public function __construct(string $fullname = null, int $age = null) {
$this->fullname = $fullname;
$this->age = $age;
}
public function printDetails() {
echo "Name : " . $this->fullname . "<br>Age : " . $this->age;
}
}
//create object of type Person with arguments passed for constructor
$person1 = new Person("Abc Defg", 24);
//try to access protected property of class
echo $person1->fullname;
?>
Output
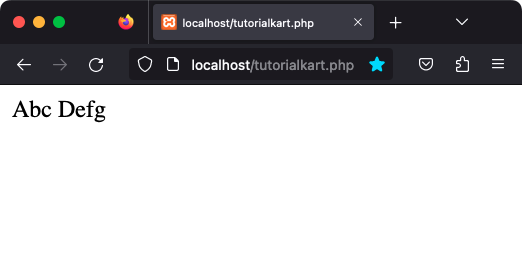
Conclusion
In this PHP Tutorial, we learned how to solve a PHP fatal error Uncaught Error: Cannot access protected property with two possible solutions.