In this tutorial, you shall learn about PHP array_diff_ukey() function which can compute the difference of an array against other arrays based on keys where comparison of keys is done based on user defined callback function, with syntax and examples.
PHP array_diff_ukey() Function
PHP array_diff_ukey() function computes the difference of an array against other arrays based on keys. The logic for comparison of keys is done using user defined callback function.
In this tutorial, we will learn the syntax of array_diff_ukey(), and how to use this function to find the difference of an array from other arrays based on keys, covering different scenarios based on array type and arguments.
Syntax of array_diff_ukey()
The syntax of PHP array_diff_ukey() function is
array_diff_ukey ( array $array1 , array $array2 [, array $... ], callable $key_compare_func ) : array
where
Parameter | Description |
---|---|
array1 | [mandatory] Array of interest. Compare the keys/index of this array against other arrays’. |
array2 | [mandatory] Array of reference. The keys of this array are used to comparison against. |
… | [optional] More arrays, along with array2, to compare against. |
key_compare_func | This function must return an integer: 1. less than zero, if first argument is less than second argument. 2. equal to zero, if first argument equals second argument. 3. greater than zero, if first argument is greater than second argument. |
Return Value
The array_diff_ukey() function returns an array of elements whose keys are present in $array1 but not present in $array2 or other arrays(if provided).
Examples
1. Compute difference of Arrays (based on Keys)
In this example, we will take an associative array, array1, with key-value pairs; compare it against another array, array2; and find their difference using array_diff_ukey() function.
PHP Program
<?php
function key_compare_func($a, $b) {
if ($a === $b) {
return 0;
}
return ($a > $b)? 1:-1;
}
$array1 = array('a'=>'apple', 'b'=>'banana', 'c'=>'cherry');
$array2 = array('a'=>'avocado', 'c'=>'citrus');
$result = array_diff_ukey($array1, $array2, "key_compare_func");
print_r($result);
?>
Output
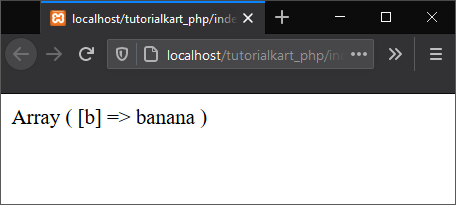
The keys 'a'
and 'c'
of array1 are present in array2. But key 'b'
of array1 is not present in array2. array_diff_ukey() returns array with key-value pairs, whose keys are present in array1, but not in array2. Therefore, the resulting array has key-value pair with key 'b'
only.
'b'=>'banana'
and 'b1'=>'banana'
differ in the key part.
2. Compute difference of Array against Multiple Arrays
In this example, we will take an array: array1 and find the difference of this array against two arrays: array2 and array3, using array_diff_ukey() function.
PHP Program
<?php
function key_compare_func($a, $b) {
if ($a === $b) {
return 0;
}
return ($a > $b)? 1:-1;
}
$array1 = array('a'=>'apple', 'b'=>'banana', 'c'=>'cherry');
$array2 = array('a'=>'avocado');
$array3 = array('c'=>'citrus');
$result = array_diff_ukey($array1, $array2, $array3, "key_compare_func");
print_r($result);
?>
Output
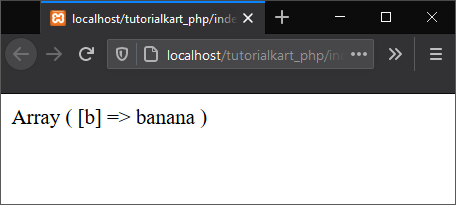
The key 'a'
is present in array2, and the key 'c'
is present in array3. Rest of the keys of array1 are not present in any of the arrays [array2, array3]. So, those key-value pairs of array1, that are not present in other arrays are returned in the resulting array.
Conclusion
In this PHP Tutorial, we learned how to compute the difference of arrays considering only key/index, using PHP Array array_diff_ukey() function.