In this tutorial, you shall learn how to define a read only property in a class in PHP, with the help of example programs.
PHP – Readonly Property in Class
We can declare the property as readonly property by writing the readonly
keyword before the datatype in the property declaration.
Once the property is declared readonly, it can be initialized only once, and that too from the scope where it has been declared, like through a constructor, or a method.
Once a readonly property is initialised, we cannot reassign a new value to this property.
In this tutorial, we declare a property as readonly and go through the process of initialising it through a constructor or a method.
Syntax
The syntax to declare a property $fullname
, in Person
class, as readonly is
class Person() {
public readonly string $fullname;
}
Examples
1. Initialize readonly property using constructor
In this example we take a class Person
and declare the property $fullname
as readonly. We initialise this read only property in the constructor.
PHP Program
<?php
class Person {
public readonly string $fullname;
public function __construct(string $fullname) {
$this->fullname = $fullname;
}
}
//create object
$person1 = new Person("Abc Defg");
//access read only property
echo $person1->fullname;
?>
Output
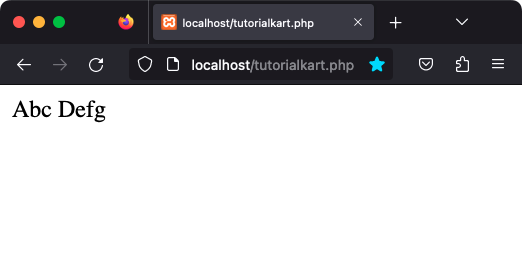
As we already mentioned, once a readonly property is initialised, we cannot reassign a value to this property.
Now let us try to reassign a value to the already initialised readonly property and observe the error.
PHP Program
<?php
class Person {
public readonly string $fullname;
public function __construct(string $fullname) {
$this->fullname = $fullname;
}
}
//create object
$person1 = new Person("Abc Defg");
//trying to assign a new value to readonly property
$person1->fullname = "Xyz Pqr";
//access read only property
echo $person1->fullname;
?>
Output
[01-Mar-2023 02:24:11 Europe/Berlin] PHP Fatal error: Uncaught Error: Cannot modify readonly property Person::$fullname in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php:13
Stack trace:
#0 {main}
thrown in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php on line 13
2. Initialize readonly property using a class method
Read only property can be initialised within the scope. Therefore, the class method can initialiser air read only property.
In the following programme, we initialise the readonly property $fullname
from the class method setFullname()
.
PHP Program
<?php
class Person {
public readonly string $fullname;
public function setFullname(string $fullname) {
$this->fullname = $fullname;
}
}
//create object
$person1 = new Person();
$person1->setFullname("Abc Defg");
//access read only property
echo $person1->fullname;
?>
Output
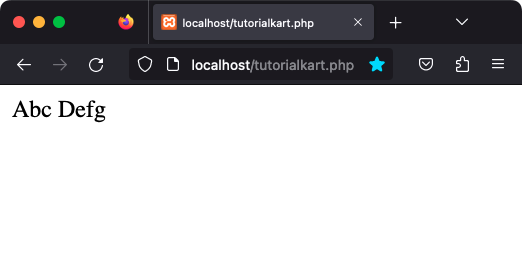
Conclusion
In this PHP Tutorial, we learned how to declare a readonly property in a class, with examples.