In this PHP tutorial, you shall learn how to find the substring of a given string using substr() function, with example programs.
PHP – Find substring of a string
To find substring of a string in PHP, we can use substr() function. Call substr() function, and pass the original given string, starting index, and substring length as arguments.
Syntax
The syntax of substr()
function is
substr(str, start, length)
str
is the given string.start
is the starting position of substring in the stringstr
.length
is the length of substring in the string. This is an optional parameter.
The function returns the substring.
If length is not specified, then the substring is considered till the end of the original string str
.
Examples
1. Find substring with start=3
In the following program, we take a string str
, and find the substring from starting index of 3
. We do not specify the length
parameter. The resulting substring is from the index of 3 to the end of string str
.
PHP Program
<?php $str = 'HelloWorld'; $start = 3; $output = substr($str, $start); echo 'Input : ' . $str . '<br>'; echo 'Output : ' . $output; ?>
Output
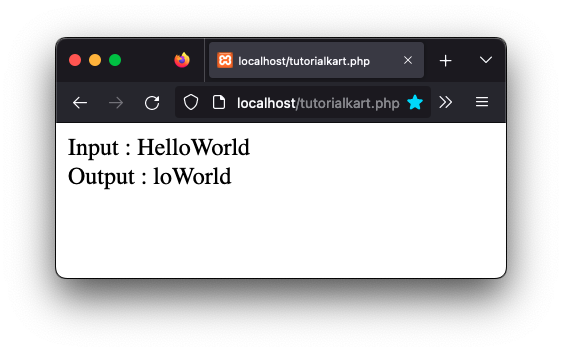
2. Find substring with start=3, and length=5
In the following program, we take a string str
, and find the substring from starting index of 3
and a length of 5.
PHP Program
<?php $str = 'HelloWorld'; $start = 3; $length = 5; $output = substr($str, $start, $length); echo 'Input : ' . $str . '<br>'; echo 'Output : ' . $output; ?>
Output
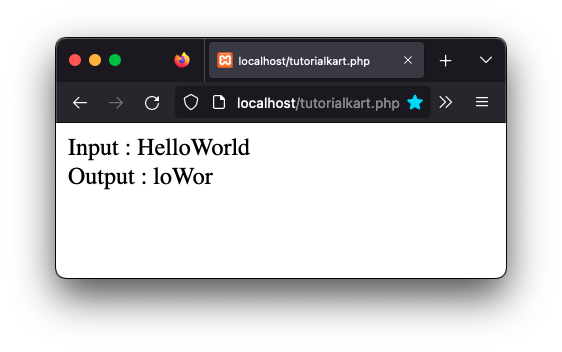
Conclusion
In this PHP Tutorial, we learned how to find substring of a string using substr()
function, with examples.