In this tutorial, you shall learn how to count the number of occurrences of a specific value in given array in PHP using count() and array_filter() functions, with the help of example programs.
PHP – Count occurrences of specific value in array
To count the number of occurrences of a specific value in given array in PHP, we can use count()
and array_filter()
functions.
array_filter()
function is used to filter only the elements that match given value, and then count()
is used to find the number of values in the filtered array.
The expression to get the count of specific value $value
in array $arr
is
count(array_filter($arr, function ($item) { return $item == $value; }))
Example
In this example, we take an array arr
with some string values. We count the occurrences of the value "apple"
in the array.
PHP Program
<?php
$arr = ["apple", "banana", "apple", "fig", "cherry"];
$value = "apple";
$n = count(array_filter($arr, function ($item) use ($value) {
return $item == $value;
}));
print_r("Number of occurrences : " . $n);
?>
Output
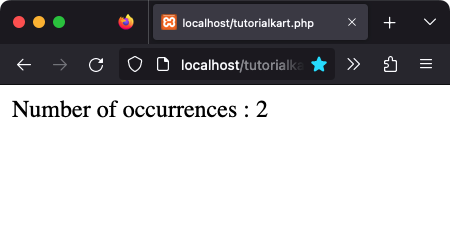
Conclusion
In this PHP Tutorial, we learned how to count the number of occurrences of a specific value in given array using count()
and array_filter()
functions.