In this tutorial, you shall learn how to create a string array in PHP, how to echo the string array to browser, how to iterate over individual strings of the array, etc.
PHP String Array
PHP String Array is an array in which the elements are strings.
Create String Array
You can use array() function with comma strings passed as argument to it, to create an array of strings. The syntax is
$arr = array(
string1,
string2,
string3
);
In the following program, we will create an array containing strings as elements.
PHP Program
<?php
$arr = array(
"apple",
"banana",
"cherry"
);
?>
You can use create an empty array first using array() function and then assign strings using offset. The syntax is
$arr = array();
$arr[0] = "string1";
$arr[1] = "string2";
In the following program, we will create an empty array and then assign strings as elements to it using index/offset.
PHP Program
<?php
$arr = array();
$arr[0] = "apple";
$arr[1] = "banana";
$arr[2] = "cherry";
?>
Echo String Array
You can use echo construct and impode() function to display all elements of string array to browser.
In the following example, we will display all elements of string array, separated by a single space.
PHP Program
<?php
$arr = array(
"apple",
"banana",
"cherry"
);
$arrString = implode(" ", $arr);
echo $arrString;
?>
Program Output
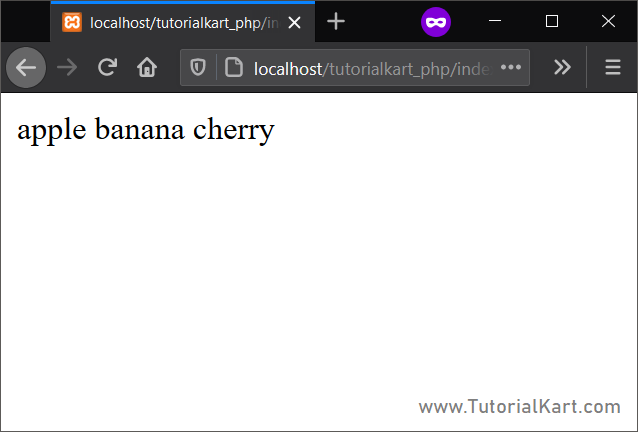
Iterate over elements of String Array
To iterate over individual strings of string array, you can use foreach loop, for loop, while loop, etc.
In the following example, we will use PHP foreach loop to iterate over elements of string array.
PHP Program
<?php
$arr = array(
"apple",
"banana",
"cherry"
);
foreach ($arr as $string) {
echo $string;
}
?>
In the following example, we will use PHP For Loop to iterate over elements of string array.
PHP Program
<?php
$arr = array(
"apple",
"banana",
"cherry"
);
for ($i = 0; $i < count($arr); $i++) {
echo $arr[$i];
}
?>
In the following example, we will use PHP While Loop to iterate over elements of string array.
PHP Program
<?php
$arr = array(
"apple",
"banana",
"cherry"
);
$i = 0;
while ( $i < count($arr) ) {
echo $arr[$i];
$i++;
}
?>
Conclusion
In this PHP Tutorial, we learned about String Arrays in PHP: how to create them, how to echo them and how to iterate over the strings in the array.