In this tutorial, you shall be introduced to Arrays in PHP, what Indexed arrays and Associative arrays are, and some examples.
PHP Arrays
An array is a collection of multiple values. The values are stored in an order.
There are two kinds of arrays in PHP.
- Indexed Arrays PHP Tutorial to learn more about indexed arrays.
- Associative Arrays PHP Tutorial to learn more about indexed arrays.
PHP Indexed Arrays
Indexed Arrays are arrays where the elements are indexed (automatically).
The following is a simple example of an integer array.
array(44, 27, 80, 61, 76, 20)
The indices of this array start at 0 for the first element, and increment by one for each subsequent element.
array(44, 27, 80, 61, 76, 20) 0 1 2 3 4 5 <--- indices
We can access individual elements using this index.
For example, if $x
is an array of four elements, $x[0]
returns the first element, $x[1]
returns the second element, and so on.
In the following example, we create an indexed array with integer values as elements, and access the individual elements using index.
PHP Program
<?php $x = array(44, 27, 80, 61, 76, 20); echo $x[0] . "<br>"; echo $x[1] . "<br>"; echo $x[2] . "<br>"; echo $x[3] . "<br>"; echo $x[4] . "<br>"; echo $x[5] . "<br>"; ?>
Output
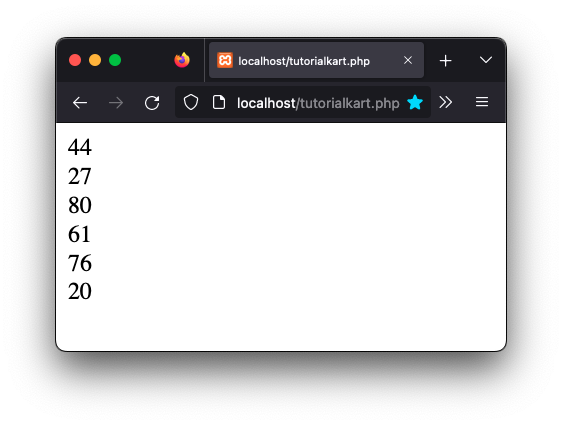
PHP Associative Arrays
Associative Arrays are arrays where the each element is a key-value pair.
The following is a simple example of an associative array.
array('apple'=>44, 'banana'=>27, 'cherry'=>80)
In the element 'apple'=>44
, 'apple'
is the key and 44
is the value.
We can access individual elements using the respective keys.
For example, if $x
is an associative array, then $x['apple']
returns the value corresponding to the key 'apple'
.
In the following example, we create an associative array with string keys and integer values, and access the individual elements using keys.
PHP Program
<?php $x = array('apple'=>44, 'banana'=>27, 'cherry'=>80); echo $x['apple'] . "<br>"; echo $x['banana'] . "<br>"; echo $x['cherry'] . "<br>"; ?>
Output
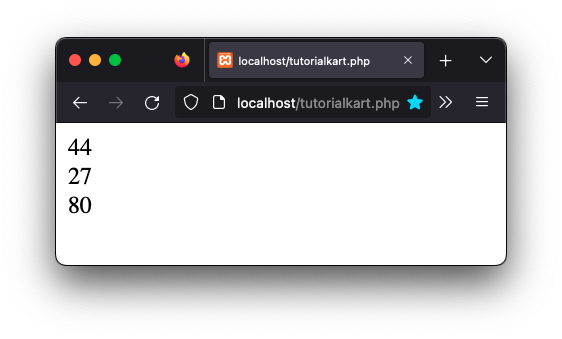
Conclusion
In this PHP Tutorial, we learned about Arrays in PHP, how to create arrays, and how to access individual elements using index or keys, with the help of examples.