In this PHP tutorial, you shall learn to use foreach statement wit array to execute a block of statements for each element in the array, with examples.
PHP Array foreach
In PHP, foreach is a construct that allows to iterate over arrays easily.
We shall go through the following scenarios, with programs.
- Iterate over array of numbers using foreach.
- Iterate over key-value pairs of associative array using foreach.
- Iterate over only values of PHP array with key-value pairs using foreach.
Syntax
The syntax of foreach statement to iterate over the items in an array $arr
is
foreach ($array as $value) {
//statement(s)
}
Using this syntax, you can iterate over arrays containing just the values as elements, or iterate only over values of an array with key-value pairs.
If you would like to access both key and value of key-value pairs in array with foreach, use the following syntax.
foreach ($arr as $key => $value) {
//statement(s)
}
Now, you can access both key and value using $key
and $value
variables respectively.
Examples (3)
1. Iterate over Array of Integers using foreach
In the following program, we take an array of integers and iterate over the elements using foreach loop.
PHP Program
<?php
$arr = array( 41, 96, 65 );
foreach ($arr as $value) {
echo $value;
echo '<br>';
}
?>
Output
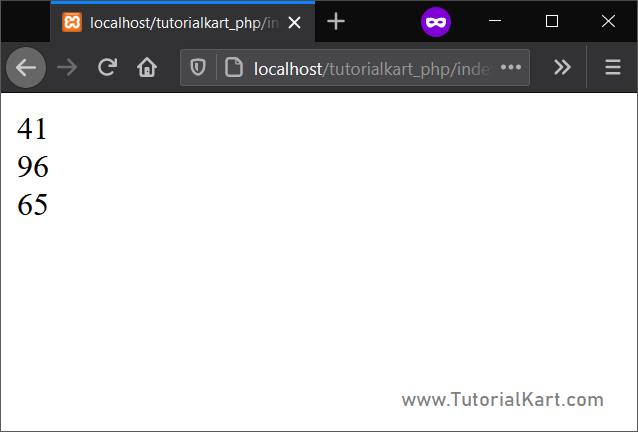
2. Iterate over key-value pairs of array using foreach
In the following program, we take an array of integers and iterate over the elements using foreach loop.
PHP Program
<?php
$arr = array(
"a" => 41,
"b" => 96,
"c" => 65
);
foreach ($arr as $key => $value) {
echo $key . ' - ' . $value;
echo '<br>';
}
?>
Output
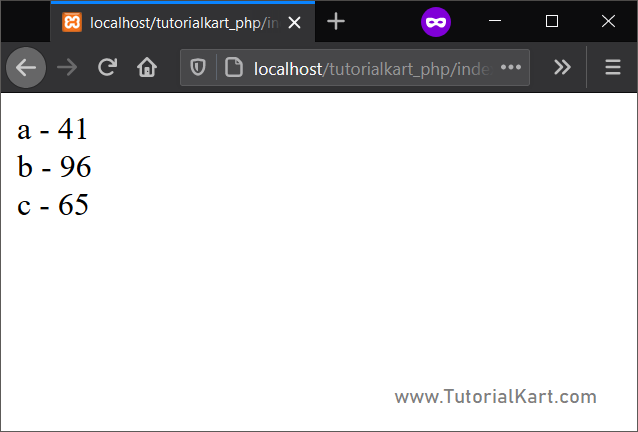
3. Iterate over Values of Associative Array
In the following program, we take an array of key-value pairs and iterate over only values using foreach loop.
PHP Program
<?php
$arr = array(
"a" => 41,
"b" => 96,
"c" => 65
);
foreach ($arr as $value) {
echo $value;
echo '<br>';
}
?>
Output
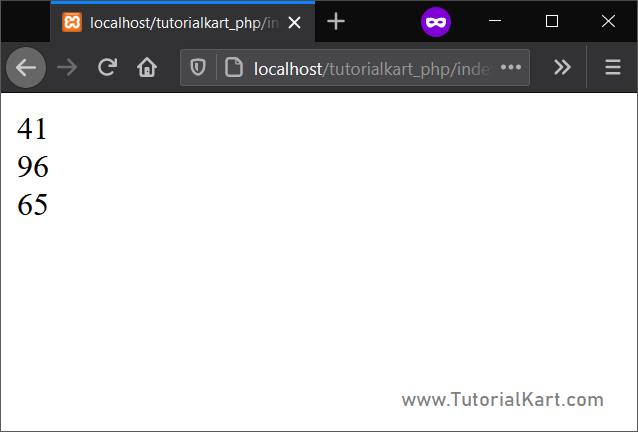
Conclusion
In this PHP Tutorial, we learned the syntax of foreach in PHP, and how to use foreach to iterate over the elements of an array.