In this tutorial, you shall learn how to define a custom exception in PHP, with the help of example programs.
PHP – User defined / Custom Exception
In PHP, we can create user defined exception (a class) by extending it with the built-in Exception class.
Syntax
The syntax to create a simple user defined exception MyException
is given below.
class MyException extends Exception { }
If required, we can define constructor and custom functions for this class.
Examples (2)
1. A simple program that defines a custom Exception
In the following program, we define a custom exception, MyException
and throw this exception from try-block. We shall catch this exception in catch-block and print the exception message to output using echo statement.
PHP Program
<?php
class MyException extends Exception { }
try {
//some code
throw new MyException("This is a message from MyException.");
} catch (MyException $e) {
//handle exception
echo $e->getMessage();
}
?>
Output
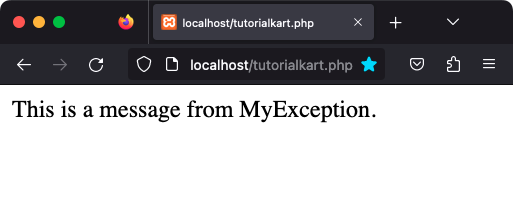
References
2. Custom exception with constructor and custom function
In the following program, we define a custom exception, MyException
and define a constructor __construct()
and a custom function myFunction()
for this class.
Note: Please note to make an exclusive call to the constructor of the superclass Exception.
PHP Program
<?php
class MyException extends Exception {
public function __construct($message, $code = 0, Throwable $previous = null) {
// some code
// call the constructor of Exception
parent::__construct($message, $code, $previous);
}
public function myFunction() {
echo "A custom function in MyException.";
}
}
try {
//some code
throw new MyException("This is a message from MyException.");
} catch (MyException $e) {
//handle exception
echo $e->getMessage();
echo "<br>";
echo $e->myFunction();
}
?>
Output
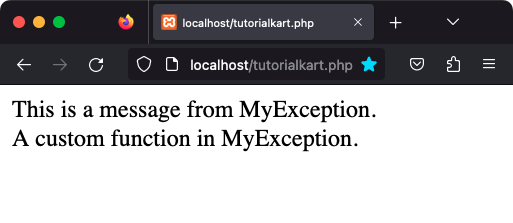
References
Conclusion
In this PHP Tutorial, we learned how to create a custom exception class and throw this custom exception object in a try-catch statement.