In this PHP tutorial, you shall learn about foreach statement, its syntax, and how to use foreach statement to iterate over elements of a collection, example programs.
PHP foreach
PHP foreach looping statement executes a block of statements once for each of the element in the iterable (or iterable expression).
The iterable expression could be an array, or an Iterable.
Syntax
The syntax of foreach statement is
foreach (iterable_expression as $element) {
statement(s)
}
The above syntax can be read as “for each element in the iterable_expression accessed as $element, execute statement(s)“.
During each iteration of the foreach loop, you can access this element of the iterable for that iteration, via $element
variable. You may change the variable name as it is developer’s choice.
For associative arrays where elements are key-value pairs, the syntax of foreach statement is
foreach ($array as $key=>$value) {
statement(s)
}
You can access the key and value during each iteration using the variables $key and $value.
Examples (3)
1. foreach with Array
In this example, we will take an indexed array of elements, iterate over each of the elements in the array, and execute a block of code (or statements) for each element.
Inside foreach block, we will print square of the element.
PHP Program
<?php
$array = [2, 5, 3, 7, 1, 8];
foreach ( $array as $element ) {
echo $element * $element;
echo "<br>";
}
?>
Output
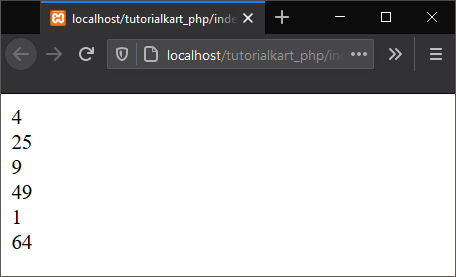
2. foreach with Iterable
In this example, we will take a generator function x()
that returns an iterable. x() can be used as an iterable_expression
in the syntax of foreach statement.
PHP Program
<?php
function x(): iterable {
yield "Apple";
yield "Banana";
yield "Cherry";
}
foreach (x() as $item) {
echo $item;
echo '<br>';
}
?>
Output
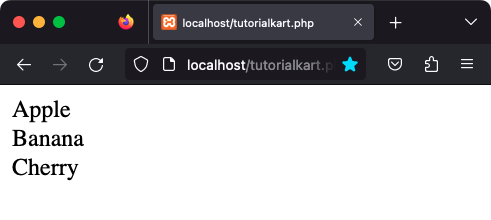
3. foreach with Associative Array
In this example, we will take an associative array of key-value pairs, and iterate over each of the key-value pairs in the array, and execute a block of code (or statements) for each key-value pair.
Inside foreach block, we will print the key and value.
PHP Program
<?php
$array = ["mango"=>2, "apple"=>5, "orange"=>3];
foreach ( $array as $key=>$value ) {
echo $key . " - " . $value;
echo "<br>";
}
?>
Output
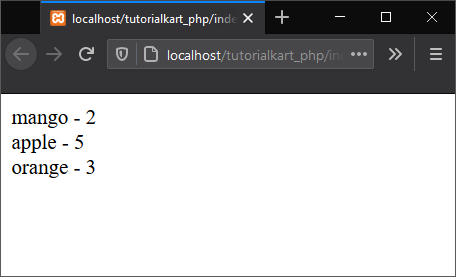
More Tutorials
The following PHP tutorials cover some of the scenarios that use foreach statement.
- PHP Array foreach PHP Tutorial to use foreach statement on arrays covering more examples.
- PHP Nested foreach PHP Tutorial to nest foreach statements for traversing multidimensional arrays.
- PHP – Iterate over key-value pairs of array PHP Tutorial to iterate over key-value pairs of associative array using foreach statement.
Conclusion
In this PHP Tutorial, we learned what foreach statement is in PHP, how to use it to traverse elements of arrays or iterables, and how to nest foreach to access elements of multi-dimensional arrays.