In this tutorial, you shall learn how to check if given two strings are equal in PHP using Equal-to operator, with example programs.
PHP – Check if strings are equal
To check if two given strings are equal in PHP, use Equal-to operator and pass the two strings as operands.
If both the strings are equal in value, then Equal-to operator returns a boolean value of true
, else it returns false
.
The boolean expression to check if two strings $x
and $y
are equal is
$x == $y
This expression can be used in a conditional statement like if, if-else, etc.
Examples
1. Positive Scenario (Equal strings)
In the following example, we take string values: $x
and $y
, and check if they are equal.
We have take the string values such that they are of equal value. Therefore, based on the program and given values, if-block must run.
PHP Program
<?php $x = "apple"; $y = "apple"; if ( $x == $y ) { echo "strings are equal"; } else { echo "strings are not equal"; } ?>
Output
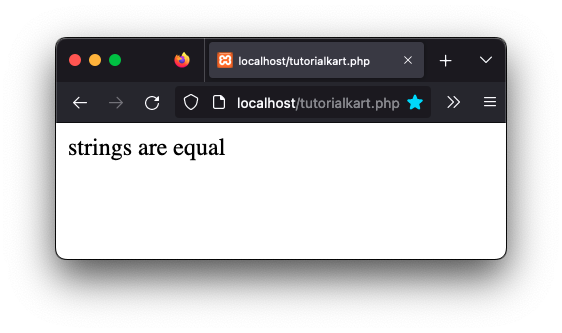
2. Negative Scenario (Not equal strings)
In the following example, we take different string values in $x and $y, and check if they are equal.
Since the string values are not equal in value, else-block must run.
PHP Program
<?php $x = "apple"; $y = "banana"; if ( $x == $y ) { echo "strings are equal"; } else { echo "strings are not equal"; } ?>
Output
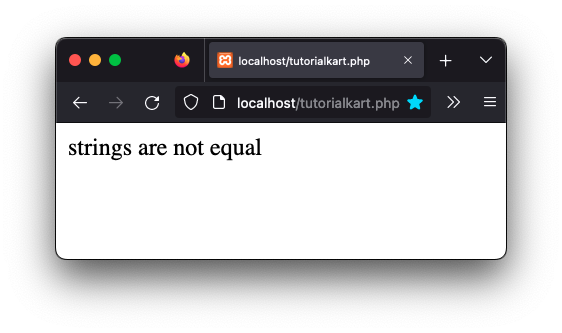
Conclusion
In this PHP Tutorial, we learned how to check if two given strings are equal or not, with the help of example programs.