In this PHP tutorial, you shall learn how to check if given string ends with a specific string using substr_compare() function, with example programs.
PHP – Check if String Ends with Substring
To check if string ends with specific substring, use substr_compare() function to compare the substring of the given string from a specific position, with the specific substring.
Step by step process
Step by step process to check if strings ends with a specific substring is
- Take string in variable $string.
- Take substring in $substring.
- Compute the length of $substring and store it in $length.
- Call substr_compare() function with the $string, $substring and negative $length passed as arguments. Negative length tells substr_compare() function to take the last part of the given string for a length given by $length.
- If given string ends with the specific substring, substr_compare() returns 0. Write this as a condition in PHP If statement.
ADVERTISEMENT
Examples
1. Check if string ends with “world”
In this example, we will implement the steps mentioned above in PHP language.
PHP Program
<?php $string = "hello world"; $substring = "world"; $length = strlen($substring); if ( substr_compare($string, $substring, -$length) === 0 ) { echo "\"{$string}\" ends with \"{$substring}\"."; } else { echo "\"{$string}\" does not end with \"{$substring}\"."; } ?>
Output
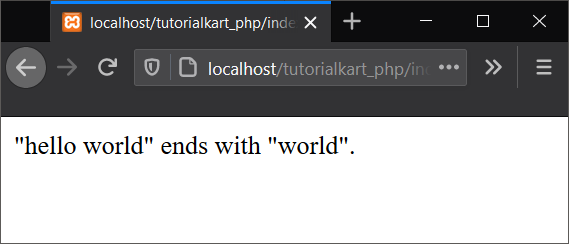
Conclusion
In this PHP Tutorial, we learned how to check if a string ends with a specific substring, using PHP built-in function substr_compare().