In this tutorial, you shall learn how to slice an array by index in PHP using array_slice() function, with example programs.
PHP – Slice an array by index
To slice an array by index in PHP, you can use array_slice() function.
Call array_slice() function, and pass the string, the offset index, and an optional slice length as arguments. The function returns the slice from the array defined by the given offset index and optional slice length.
array_slice($array, $offset) array_slice($array, $offset, $length)
Examples
1. Slice from specific offset index to end of the array
In the following program, we take an array $arr
, and slice it from an offset index of 2
till the end of the array using array_slice() function.
PHP Program
<?php $array = array('a', 'b', 'c', 'd', 'e', 'f', 'g'); $offset = 2; $sliced_array = array_slice($array, $offset); print_r($sliced_array); ?>
Output
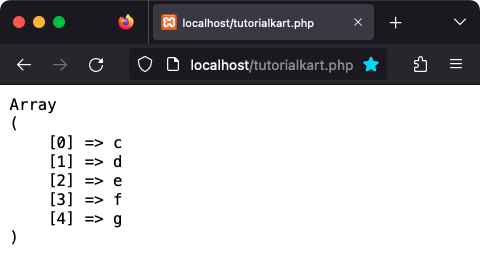
If you observe the keys, they have been reset. But, if you would like to preserve the keys, we can pass the flag as an argument to the array_slice() function as shown in the following program.
PHP Program
<?php $array = array('a', 'b', 'c', 'd', 'e', 'f', 'g'); $offset = 2; $sliced_array = array_slice($array, $offset, null, true); print_r($sliced_array); ?>
Output
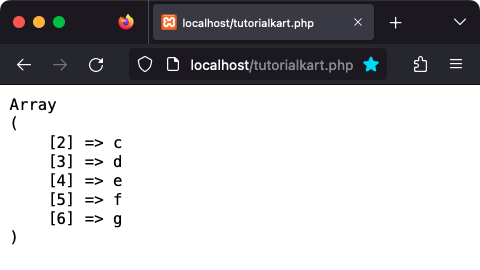
2. Slice array from specific offset index for a specific length
In the following program, we take an array $arr
, and slice it from an offset index of 2
for a length of 3
using array_slice() function.
PHP Program
<?php $array = array('a', 'b', 'c', 'd', 'e', 'f', 'g'); $offset = 2; $length = 3; $sliced_array = array_slice($array, $offset, $length); print_r($sliced_array); ?>
Output
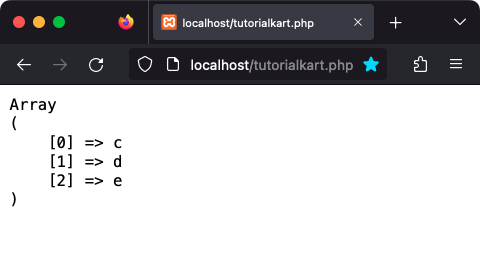
Conclusion
In this PHP Tutorial, we learned how to slice an array by index, using array_slice() function.