In this tutorial, you shall learn how to check if the entire string matches a given regular expression in PHP using preg_match() function, with the help of example programs.
PHP – Check if entire string matches a regular expression
To check if the entire string matches a given regular expression in PHP, we can use preg_match() function.
Call the preg_match() function and pass the pattern, and input string as arguments.
Please note that the function preg_match() checks only if there is a match for the regular expression in the given string. Therefore, we have to specify in the pattern, to match the starting and ending of the given string, to check if the entire string matches the regular expression.
Syntax
The syntax to call preg_match() function to check if the entire string $str
matches the regular expression in the pattern $pattern
is
preg_match($pattern, $str)
If the string $str
matches the regular expression in the $pattern
, then the function returns 1
. If there is no match, then the function returns 0
. Or else in case of any error, a boolean value of false is returned.
Examples
1. Check if given string is a valid name of a person
In this example, we take the name of a person as an input string in str
and take a pattern that validates if given string is a valid name or not.
Let us consider that the given name is valid if the entire string matches the pattern of word followed by a word separated by a space, where the word contains only alphabets.
PHP Program
<?php
$str = "Alex Burger";
$pattern = "/^([a-z]+)\s([a-z]+)$/i";
if(preg_match($pattern, $str)) {
echo "Given string is a valid name.";
} else {
echo "Given string is not a valid name.";
}
?>
Output
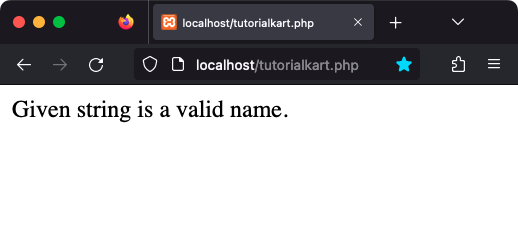
Now, let us take an input string that is not a valid name.
PHP Program
<?php
$str = "Alex 123Burger";
$pattern = "/^([a-z]+)\s([a-z]+)$/i";
if(preg_match($pattern, $str)) {
echo "Given string is a valid name.";
} else {
echo "Given string is not a valid name.";
}
?>
Output
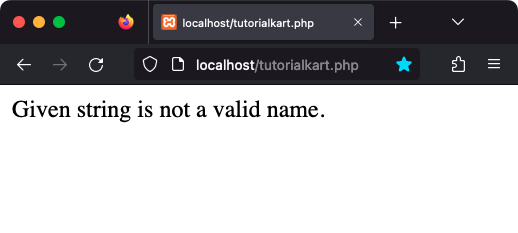
Reference to tutorials related to program
Conclusion
In this PHP Tutorial, we learned how to check if the entire string matches a given pattern or not using preg_match() function, with the help of examples.