In this tutorial, you shall learn how to convert a string to a boolean value in PHP using Identity operator, with the help of example programs.
PHP – Convert string to boolean
Let us consider a scenario where we have a Boolean value of true or false as a string: "true"
or "false"
. And we need to convert this string value to a boolean type value in PHP.
To convert the string value to a boolean in PHP, convert the string to lower case and check if this equals true or false using PHP If statement.
Example
In the following program, we take a string value in variable $x, and convert this string value into a Boolean value.
We have considered that the default value of converted Boolean value $bool
is false.
PHP Program
<?php $str = 'TRUE'; $bool = false; if (strtolower($str) === "true") { $bool = true; } echo "Boolean value : " . $bool; ?>
Output
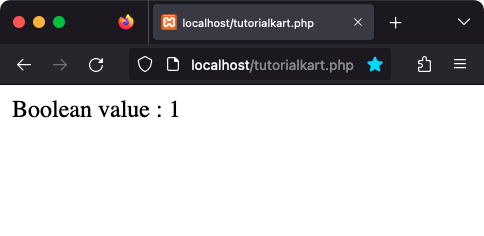
Now let us change the value of input string $str
to "False"
and check the output.
PHP Program
<?php $str = 'False'; $bool = false; if (strtolower($str) === "true") { $bool = true; } echo "Boolean value : " . $bool; ?>
Output
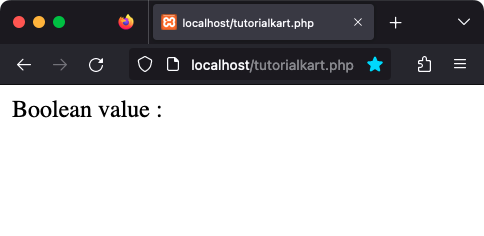
Reference tutorials for the program
Conclusion
In this PHP Tutorial, we learned how to convert a string value of "true"
or "false"
, ignoring the case, to a boolean value.