In this tutorial, you shall learn about PHP array_column() function which can get the values from a single column in the input array, with syntax and examples.
PHP array_column() Function
PHP array_column() function returns the values from a single column in the input array, where you specify the column with a column key.
In this tutorial, we will learn the syntax of array_column(), and how to get a specific column from a two dimensional array, covering different scenarios based on array type and arguments.
Syntax of array_column()
The syntax of PHP array_column() function is
array_column ( array $input , mixed $column_key [, mixed $index_key = NULL ] ) : array
where
Parameter | Description |
---|---|
input | [mandatory] input is the array from which we get the column values. |
column_key | [mandatory] Columns in an array can be identified using integer index in indexed arrays or string key name in associative arrays. This index or string key can be given as column_key. |
index_key | [optional] index_key is used as index or keys for the returned column values. The values corresponding to this key in each row is selected as index for the column value in returned array. |
Return Value
The array_column() function returns a single column for the given input array, where the column is selected using column_key. If index_key is provided, then values of this index_key are applied as index or key to the respective column values that are returned.
Examples
1. Get values of a single column in the given array
In this example, we will take a two dimensional array with key-value pairs, and get values of a single column, identified by the key 'last_name'
.
PHP Program
<?php $input = array( array( 'id' => 42, 'first_name' => 'A', 'last_name' => 'B', ), array( 'id' => 56, 'first_name' => 'X', 'last_name' => 'Y', ), array( 'id' => 79, 'first_name' => 'P', 'last_name' => 'Q', ) ); $column_key = 'last_name'; $column_values = array_column($input, $column_key); print_r($column_values) ?>
Output
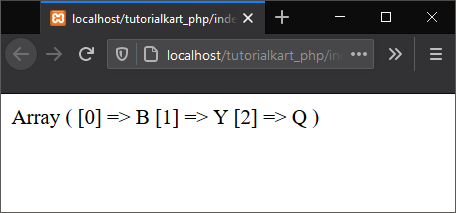
There are two observations that we can draw from this output. They are
- The values in the rows, corresponding to the column specified by key ‘c’ are returned as an array.
- The index for these values are set to default, as in an indexed array.
2. Get values of a single column in Indexed Array
In this example, we will take a two dimensional indexed array, and get values of a single column, identified by the column index 1
.
PHP Program
<?php $input = array( array(42, 'A', 'B',), array(56, 'X', 'Y',), array(79, 'P', 'Q',) ); $column_key = 1; $column_values = array_column($input, $column_key); print_r($column_values) ?>
Output
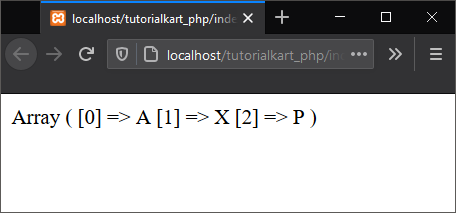
3. Get values of a single column in an array, with given index key
In this example, we will take a two dimensional array, where inner arrays are defined with key-value pairs, same as in the previous example, and then get column values with key 'last_name'
. We shall apply an index to this returned values, by passing the indexes array as third argument to the array_column() function.
PHP Program
<?php $input = array( array( 'id' => 42, 'first_name' => 'A', 'last_name' => 'B', ), array( 'id' => 56, 'first_name' => 'X', 'last_name' => 'Y', ), array( 'id' => 79, 'first_name' => 'P', 'last_name' => 'Q', ) ); $column_key = 'last_name'; $index_key = 'id'; $column_values = array_column($input, $column_key, $index_key); print_r($column_values) ?>
Output
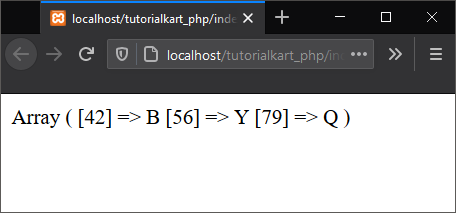
We have specified the index for column values to be 'id'
. So, for the first column value, 'B'
, index is the value for the key 'id'
in that array, which is 42
. Similarly, index for the values from other rows is also selected.
Conclusion
In this PHP Tutorial, we learned how to extract or get a single column from a given input array using PHP Array array_column() function.