In this tutorial, you shall learn about PHP array_sum() function which can find the sum of all values in the array, with syntax and examples.
PHP array_sum() Function
The PHP array_sum() function computes sum (addition) of all numbers in an array and returns the result.
If array contains items of different datatypes, only the numbers are considered for addition operation.
Syntax of array_sum()
The syntax of array_sum() function is
array_sum( array )
where
Parameter | Description |
---|---|
array | [mandatory] The array whose elements’ sum has to be computed. |
Function Return Value
array_sum() returns the sum of all numbers in an array.
Examples
1. Find the sum of numbers in an Array
In this example, we will take an array with three numbers. We will pass this array as argument to array_sum() function. The function computes the sum of numbers in the array and returns the result.
PHP Program
<?php
$array1 = array(5, 7, 2);
$result = array_sum($array1);
print_r($array1);
echo "<br>Sum is: ";
echo $result;
?>
Output
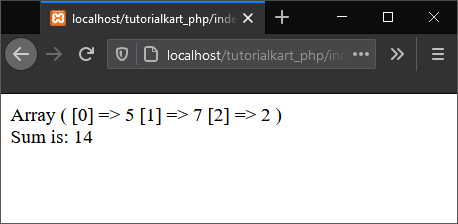
2. Sum of Floating Point Numbers in Array
In this example, we will take an array with floating point numbers. We will find the sum of these numbers in the array using array_product() function.
PHP Program
<?php
$array1 = array(5.8, 2.2, 4.8);
$result = array_sum($array1);
print_r($array1);
echo "<br>Sum is: ";
echo $result;
?>
Output
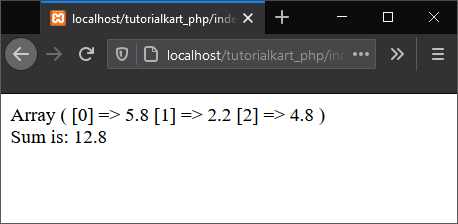
3. Find sum of items in an Array with numbers and strings
In this example, we will take an array with numbers and strings. array_sum() function considers only the numbers in the array for addition operation and ignores the rest.
PHP Program
<?php
$array1 = array(5, 7, "apple");
$result = array_sum($array1);
print_r($array1);
echo "<br>Sum is: ";
echo $result;
?>
Output
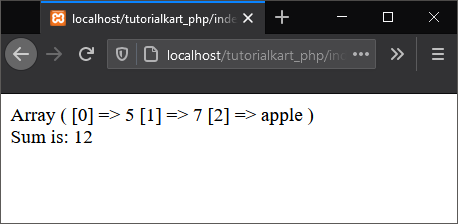
Conclusion
In this PHP Tutorial, we learned how to find the sum of numbers in an array, using PHP Array array_sum() function.