In this tutorial, you shall learn how to replace the first occurrence of a search string in a string in PHP using substr_replace() function, with the help of example programs.
PHP – Replace first occurrence in a string
To replace the first occurrence of a search string in a string with a replacement string in PHP, use substr_replace() function.
substr_replace() function can replace a substring (defined by offset index in original string, and length) in the original string with a replacement string. This function/behaviour can be used to replace the first occurrence in a string.
Syntax
The syntax of substr_replace() function to replace the first occurrence of a search string $search
with a replacement string $replacement
in the original string $input_string
is
$offset = strpos($input_string, $search); $length = strlen($search); $output_string = $input_string; //replace only if search string is present if ($offset !== false) { $output_string = substr_replace($input_string, $replacement, $offset, $length); }
First we have found the index of first occurrence of search string in the original string using strpos(). This is our offset index.
Secondly, we calculated the length of search string as $length
. This is the length of the substring which we replace in the original string.
The substr_replace() function call returns a new string created with the substring defined by the offset index and specified length replaced in the original string.
We can wrap the substr_replace() function call in PHP If statement so that we replace the substring only if the search string is present in the original string.
Examples (2)
1. Replace operation when search string is present in the string
In this example, we take an input string "apple banana apple mango"
and replace first occurrence of the search string "apple"
with the replacement string "cherry"
in this input string using substr_replace() function.
PHP Program
<?php $input_string = "apple banana apple mango"; $search = "apple"; $replacement = "cherry"; $offset = strpos($input_string, $search); $length = strlen($search); $output_string = $input_string; //replace only if search string is present if ($offset !== false) { $output_string = substr_replace($input_string, $replacement, $offset, $length); } echo "<html><pre>"; echo "Input String : " . $input_string; echo "<br>Output String : " . $output_string; echo "</pre></html>"; ?>
Output
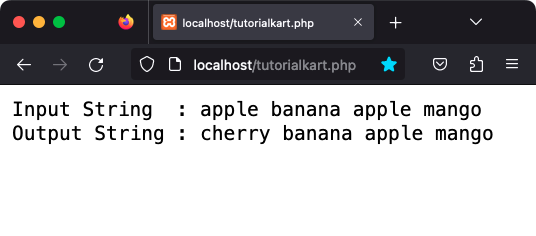
Reference tutorials for this program
2. Replace operation when search string is not present in the string
In this example, we take an input string "apple banana apple mango"
and replace the first occurrence of "guava"
with the replacement string "cherry"
in this input string.
Since the search string is not present in the original string, the value in $output_string
would be same as that of the $input_string
.
PHP Program
<?php $input_string = "apple banana apple mango"; $search = "guava"; $replacement = "cherry"; $offset = strpos($input_string, $search); $length = strlen($search); $output_string = $input_string; //replace only if search string is present if ($offset !== false) { $output_string = substr_replace($input_string, $replacement, $offset, $length); } echo "<html><pre>"; echo "Input String : " . $input_string; echo "<br>Output String : " . $output_string; echo "</pre></html>"; ?>
Output
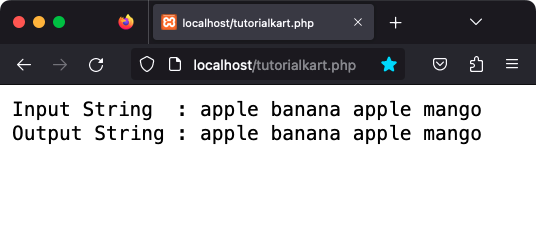
Reference tutorials for this program
Conclusion
In this PHP Tutorial, we learned how to replace the first occurrence in a string with a replacement string, using substr_replace() function.