In this tutorial, you shall learn about Arithmetic Addition Operator in PHP, its syntax, and how to use this operator in PHP programs, with examples.
PHP Addition
PHP Arithmetic Addition Operator takes two numbers as operands and returns their sum.
Symbol
+
symbol is used for Addition Operator.
Syntax
The syntax for Addition Operator is
operand_1 + operand_2
The operands could be of any numeric datatype, integer or float.
If the two operands are of different datatypes, implicit datatype promotion takes place and value of lower datatype is promoted to higher datatype.
Examples
1. Addition of integers: x + y
In the following example, we take integer values in $x
and $y
, and add them using Arithmetic Addition Operator.
PHP Program
<?php
$x = 5;
$y = 4;
$output = $x + $y;
echo "x = $x" . "<br>";
echo "y = $y" . "<br>";
echo "x + y = $output";
?>
Output
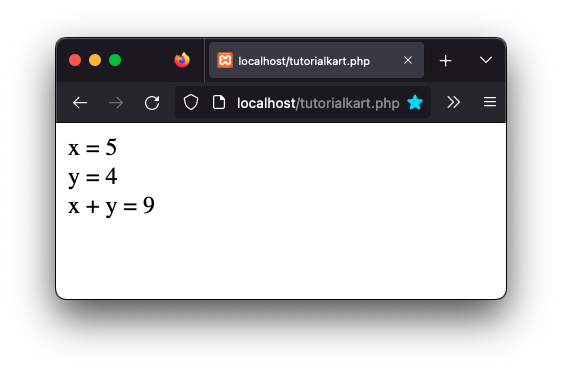
2. Addition of float values
In the following example, we take floating point values in $x
and $y
, and add them using Arithmetic Addition Operator.
PHP Program
<?php
$x = 5.1;
$y = 4.2;
$output = $x + $y;
echo "x = $x" . "<br>";
echo "y = $y" . "<br>";
echo "x + y = $output";
?>
Output
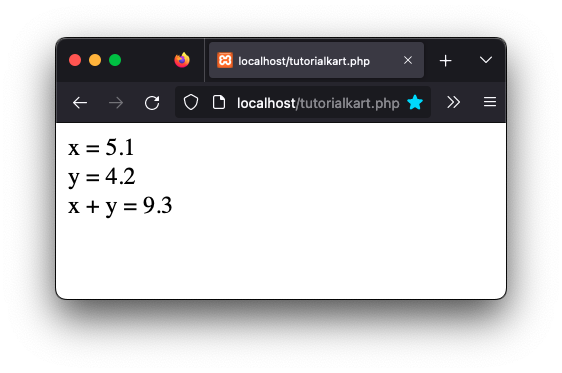
3. Addition of Integer and Float Values
In the following example, we take integer value in $x
and floating point value in $y
, and add them using Arithmetic Addition Operator.
Since float is higher datatype among integer and float, the output is float.
PHP Program
<?php
$x = 5;
$y = 4.2;
$output = $x + $y;
echo "x = $x" . "<br>";
echo "y = $y" . "<br>";
echo "x + y = $output";
?>
Output
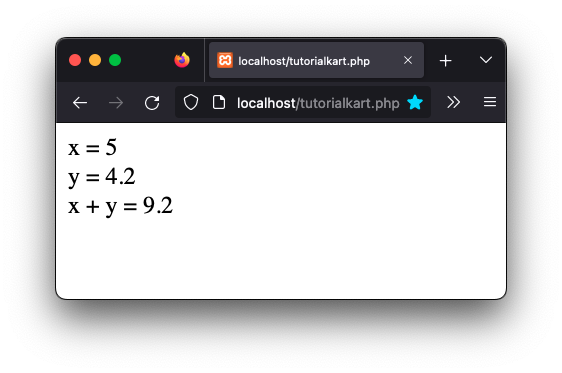
Conclusion
In this PHP Tutorial, we learned how to use Arithmetic Addition Operator to add two numbers.