In this tutorial, you shall learn about PHP array_change_key_case() function which can change the case of all keys in an array, with syntax and examples.
PHP array_change_key_case() Function
PHP array_change_key_case() function changes the case of all keys in an array.
You can mention the case, either lower or upper, as an argument to the function.
Syntax of array_change_key_case()
The syntax of PHP array_change_key_case() function is
array_change_key_case ( array $array [, int $case = CASE_LOWER ] ) : array
where
Parameter | Description |
---|---|
array | The array in which we want to change the case of keys. |
case | It takes either CASE_UPPER or CASE_LOWER. If $case = CASE_UPPER, then keys will be converted to uppercase. If $case = CASE_LOWER, then keys will be converted to lowercase. The default value is CASE_LOWER. If you do not provide an argument for case, then CASE_LOWER will be in effect. |
Return Value
The array_change_key_case() function returns a new array with its keys converted to upper or lower case, based on the case
parameter.
Examples
1. Changes the case of all keys in an array to lowercase
In this example, we will take an associative array with key-value pairs, and then change the case of all keys in the array to lower case.
PHP Program
<?php
$array = ["Mango"=>2, "Apple"=>5];
$result = array_change_key_case($array, CASE_LOWER);
print_r($result);
?>
Output
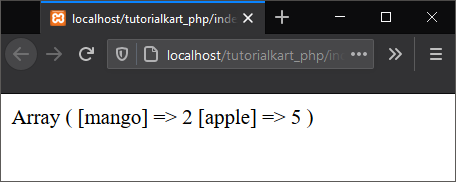
As the default value of case parameter is CASE_LOWER, we may not provide an argument value explicitly.
PHP Program
<?php
$array = ["Mango"=>2, "Apple"=>5];
$result = array_change_key_case($array);
print_r($result);
?>
Output
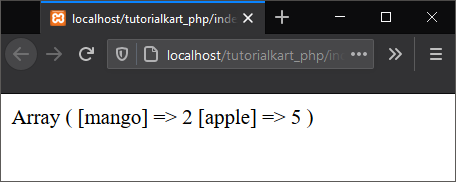
2. Change the case of all keys in an array to uppercase
In this example, we will take an associative array with key-value pairs, and then change the case of all keys in the array to upper case.
PHP Program
<?php
$array = ["Mango"=>2, "Apple"=>5];
$result = array_change_key_case($array, CASE_UPPER);
print_r($result);
?>
Output
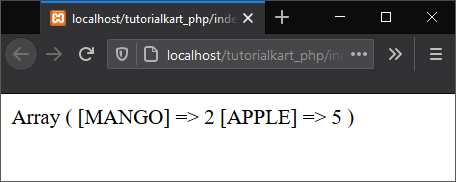
3. Warning – array_change_key_case() expects parameter 1 to be array
If the first argument to array_change_key_case() is not of type array, then the function raises a warning.
In the following example, we will pass a string to array_change_key_case() instead of an array, and observe the result.
PHP Program
<?php
$array = "Hello World!";
$result = array_change_key_case($array, CASE_UPPER);
print_r($result);
?>
Output
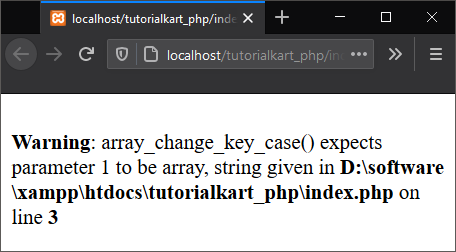
Conclusion
In this PHP Tutorial, we learned how to change the case of all keys in given array using PHP Array array_change_key_case() function.