In this tutorial, you shall learn about Arithmetic Division Operator in PHP, its syntax, and how to use this operator in PHP programs, with examples.
PHP Division
PHP Arithmetic Division Operator takes two numbers as operands and returns the quotient of the division of first operand by second operand.
Symbol
/
symbol is used for Division Operator.
Syntax
The syntax for division operator is
operand_1 / operand_2
The operands could be of any numeric datatype, integer or float.
If the two operands are of different datatypes, implicit datatype promotion takes place and value of lower datatype is promoted to higher datatype.
Examples
1. Division of Integers
In the following example, we take integer values in $x
and $y
, and find the result of division $x / $y
.
PHP Program
<?php $x = 25; $y = 4; $output = $x / $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x / y = $output"; ?>
Output
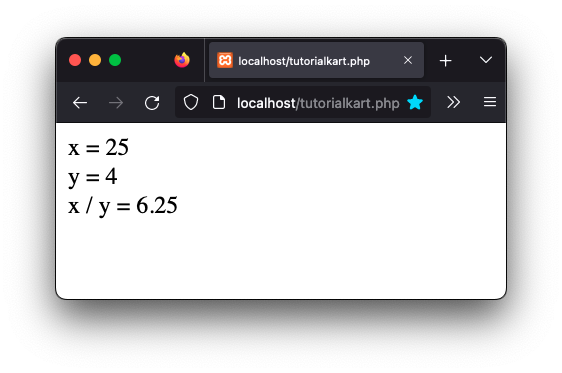
2. Division of Float Values
In the following example, we take floating point values in $x
and $y
, and find the result of division $x / $y
.
PHP Program
<?php $x = 25.6; $y = 4.1; $output = $x / $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x / y = $output"; ?>
Output
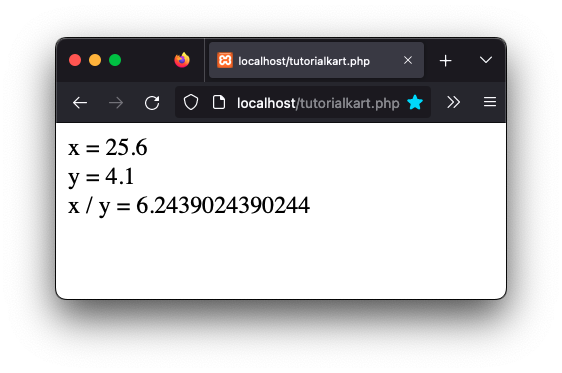
3. Division of Float by Integer
In the following example, we take integer value in $x
and floating point value in $y
, and find the division of $x
by $y
.
PHP Program
<?php $x = 25.6; $y = 4; $output = $x / $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x / y = $output"; ?>
Output
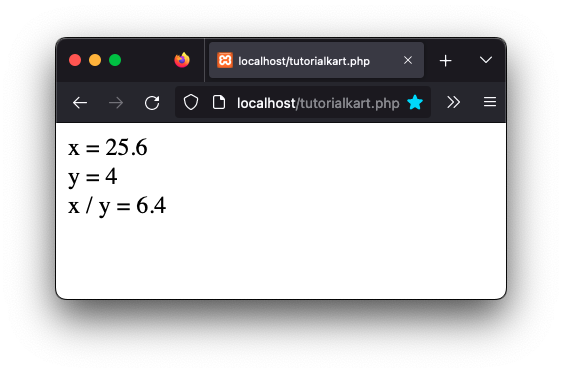
Conclusion
In this PHP Tutorial, we learned how to use Arithmetic Division Operator to find the division of a number by another.