In this tutorial, you shall learn about If conditional statement in PHP, its syntax, how to write an If conditional statement in PHP programs, and how to use it, with the help of a few example scenarios.
PHP If
PHP If statement is a conditional statement that can conditionally execute a block of statements based on the result of an expression.
Syntax
The syntax of PHP If statement is
if (condition) { // if-block statement(s) }
where
if
is a keyword.condition
is a boolean expression.if-block statement(s)
are a block of statements. if-block can contain none, one or more PHP statements.
If the condition evaluates to true, PHP executes if-block statement(s). If the condition is false, PHP does not execute if-block statement(s). After any of the two possible scenarios, PHP execution continues with the subsequent PHP statements.
Example
The following is a simple PHP program to demonstrate the working of If conditional statement.
We take two variables and assign values to each of them. Then we use if-statement to check if the two variables are equal.
Here, the condition is a equals b ?. if-block statement(s) is only a single echo statement.
PHP Program
<?php $a = 1; $b = 1; if ($a == $b) { echo "a and b are equal."; } ?>
Output
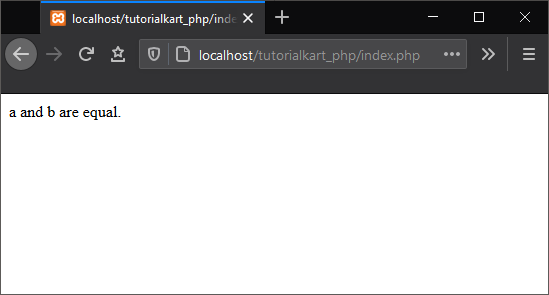
If Statement with Compound Condition
In the previous example, we have taken simple conditions with equal to operator. But, we can join these multiple simple conditions and form a compound condition to use as if condition.
- Python If statement with AND Operator
- Python If statement with OR Operator
- Python If statement with NOT Operator
In the following example, we will take following two simple conditions and combine them with logical AND operator to form a compound statement.
$a == $b
Check if a and b are equal.$a % 2 == 0
Check if a is even number.
PHP Program
<?php $a = 4; $b = 4; if ( ($a == $b) && ($a % 2 == 0) ) { echo "a and b are equal.<br>"; echo "a is divisible by 2."; } ?>
Output
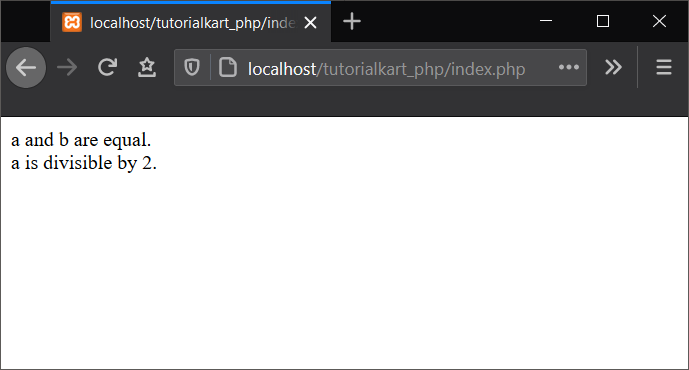
Nested If Statement
PHP If statement is just another statement. So, we can write an if-statement as part of if-block statement. This makes a nested if statement.
In the following program, we will write an if statement inside another if statement.
PHP Program
<?php $a = 4; $b = 4; if ( $a == $b ) { echo "a and b are equal.<br>"; if ( $a % 2 == 0 ) { echo "a is divisible by 2."; } } ?>
Output
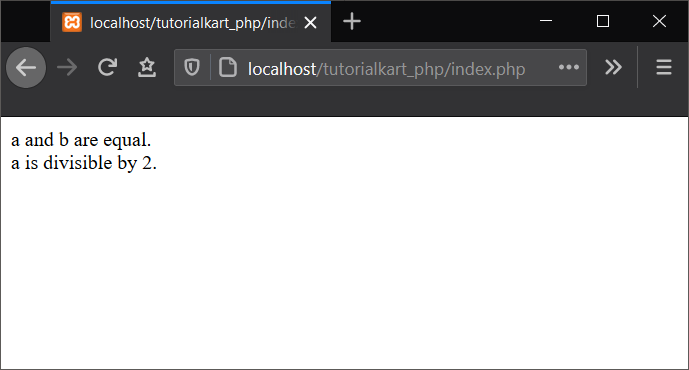
If Statement with Integer as Condition
In PHP, zero value is considered false, and a non-zero value is considered true. So, you can use integer instead of condition in if-statement.
PHP Program
<?php $a = 3; if ( $a ) { echo "a is non zero."; } ?>
Output
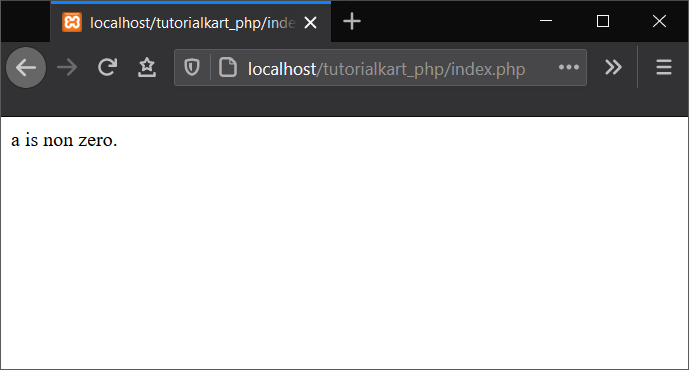
If Statement with String as Condition
In PHP, empty string is considered false, and a non-empty string is considered true. So, you can use a string instead of condition in if-statement.
PHP Program
<?php $a = "tutorialkart"; if ( $a ) { echo "a is not empty."; } ?>
Output
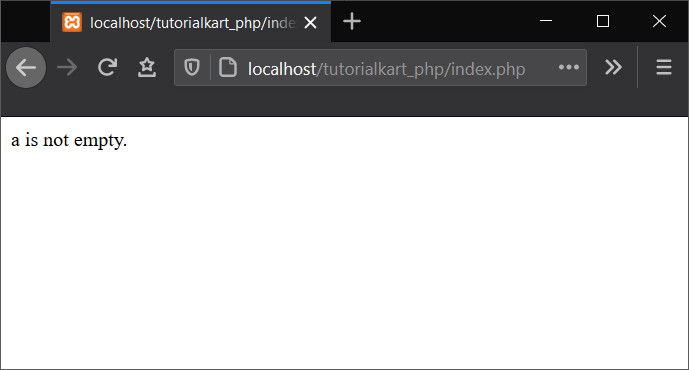
Conclusion
In this PHP Tutorial, we learned the syntax of PHP If statement, and its usage via different scenarios that we explained in detail.