In this tutorial, you shall learn how to check if two given arrays are equal in PHP using Array Identity Operator, with the help of example programs.
PHP – Check it two arrays are equal
To check if two given arrays are equal in PHP, we can use array identity operator ===
.
The array identity operator takes two arrays as operands and returns true only if the two arrays have same key/value pairs in the same order and of same types, or else it returns false.
The expression to check if arrays array1
and array2
are equal is
$array1 === $array2
If you are not worried about the order and type of values, we can use array equality operator ==
.
Examples
1. Check if array1 is equal to array2 (given equal arrays)
In this example, we take a two arrays array1
and array2
. We check if these two arrays are equal in value and order using identity operator.
PHP Program
<?php $array1 = ["apple", "banana", "cherry"]; $array2 = ["apple", "banana", "cherry"]; if( $array1 === $array2 ){ echo "Arrays are equal."; } else { echo "Arrays are not equal."; } ?>
Output
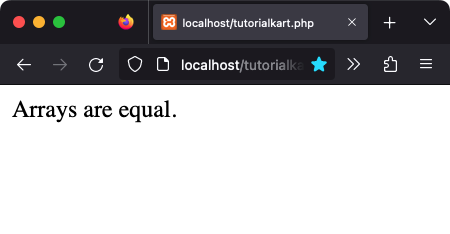
2. Check if array1 is equal to array2 (given unequal arrays)
In this example, we take a two arrays array1
and array2
such that they are not equal. We check if these two arrays are equal or not programmatically, in value and order, using identity operator.
PHP Program
<?php $array1 = ["apple", "banana", "cherry", "mango"]; $array2 = ["apple", "banana", "cherry"]; if( $array1 === $array2 ){ echo "Arrays are equal."; } else { echo "Arrays are not equal."; } ?>
Output
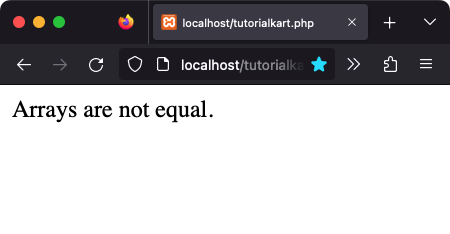
Conclusion
In this PHP Tutorial, we learned how to check if two arrays are equal using array identity operator.