In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples.
Assignment Operators
Assignment Operators are used to perform to assign a value or modified value to a variable.
Assignment Operators Table
The following table lists out all the assignment operators in PHP programming.
Operator | Symbol | Example | Description |
---|---|---|---|
Simple Assignment | = | x = 5 | Assigns value of 5 to variable x. |
Addition Assignment | += | x += y | Assigns the result of x+y to x. |
Subtraction Assignment | -= | x -= y | Assigns the result of x-y to x. |
Multiplication Assignment | *= | x *= y | Assigns the result of x*y to x. |
Division Assignment | /= | x /= y | Assigns the result of x/y to x. |
Modulus Assignment | %= | x %= y | Assigns the result of x%y to x. |
ADVERTISEMENT
Example
In the following program, we will take values in variables $x
and $y
, and perform assignment operations on these values using PHP Assignment Operators.
PHP Program
<?php // Simple Assignment $x = 5; echo "After Simple assignemnt, x = $x" . "<br>"; // Addition Assignment $x = 5; $y = 2; $x += $y; echo "After Addition assignemnt, x = $x" . "<br>"; // Subtraction Assignment $x = 5; $y = 2; $x -= $y; echo "After Subtraction assignemnt, x = $x" . "<br>"; // Multiplication Assignment $x = 5; $y = 2; $x *= $y; echo "After Multiplication assignemnt, x = $x" . "<br>"; // Division Assignment $x = 5; $y = 2; $x /= $y; echo "After Division assignemnt, x = $x" . "<br>"; // Modulus Assignment $x = 5; $y = 2; $x %= $y; echo "After Modulus assignemnt, x = $x" . "<br>"; ?>
Output
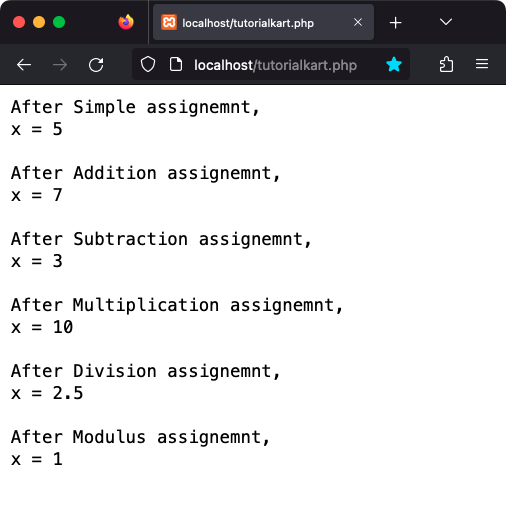
Assignment Operators Tutorials
The following tutorials cover each of the Assignment Operators in PHP in detail with examples.
- PHP Simple Assignment
- PHP Addition Assignment
- PHP Subtraction Assignment
- PHP Multiplication Assignment
- PHP Division Assignment
- PHP Modulus Assignment
Conclusion
In this PHP Tutorial, we learned about all the Assignment Operators in PHP programming, with examples.