In this tutorial, you shall learn how to slice an array by key in PHP using array_slice() function, with example programs.
PHP – Slice an array by key
To slice an array by key in PHP, you can use array_slice() function.
By default, array_slice() function slices the array by given offset index and length. But to slice an array by key, we first find the index of the key in the array, and then pass the index of the key as argument to the array_slice() function.
Refer PHP – Slice array by index tutorial. We are building on this tutorial, to find the array slice by given keys.
To get the index of a given key, we use array_search() function.
Examples
1. Slice from specific staring key to end of the array
In the following program, we take an array $arr
, and slice it from starting key of "dad"
till the end of the array using array_slice() function.
PHP Program
<?php $arr = array( 'foo' => 10, 'bar' => 20, 'das' => 30, 'qux' => 40, 'par' => 50, 'saz' => 60, ); $start_key = 'das'; //get offset of start key in the array $offset = array_search($start_key, array_keys($arr), true); $sliced_array = array_slice($arr, $offset, null, true); print_r($sliced_array); ?>
Output
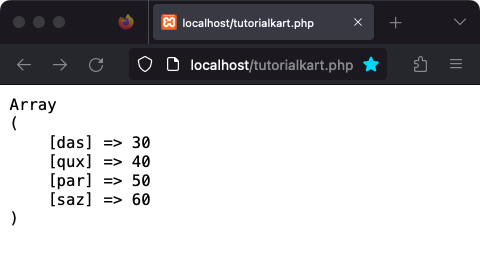
Reference tutorials for the program
2. Slice array from starting key to ending key
In the following program, we take an array $arr
, and slice it from a staring key of "das"
to the ending key of "par"
using array_slice() function.
PHP Program
<?php $arr = array( 'foo' => 10, 'bar' => 20, 'das' => 30, 'qux' => 40, 'par' => 50, 'saz' => 60, ); $start_key = 'das'; $end_key = 'par'; //get offset of start key in the array $offset = array_search($start_key, array_keys($arr), true); //get length of slice $length = array_search($end_key, array_keys($arr), true) - $offset + 1; $sliced_array = array_slice($arr, $offset, $length, true); print_r($sliced_array); ?>
Output
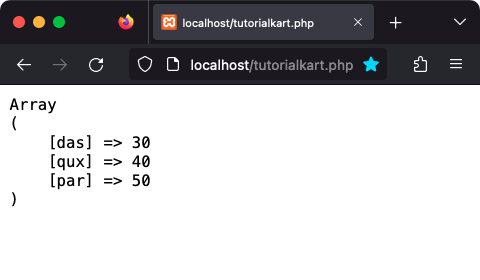
Conclusion
In this PHP Tutorial, we learned how to slice an array by key, using array_slice() function.