In this tutorial, you shall learn how to handle exceptions in PHP using try-catch statement, the syntax of try-catch statement, and how to use try-catch statement in PHP programs, with examples.
PHP – Try Catch
In PHP, try catch statement is used to handle exceptions thrown by the code.
We enclose the code that has potential to throw exceptions in try block, and address the exceptions in catch blocks.
Syntax
The syntax of try catch block is
try {
//code
} catch (Exception $e) {
//code
}
Replace Exception
with the type of exception that the try block can throw.
If there are more than one type of exception that the try block can throw, then we can have more than one catch blocks, to serve the respective exceptions.
try {
//code
} catch (Exception1 $e) {
//code
} catch (Exception2 $e) {
//code
}
Replace Exception1
and Exception2
with the type of exceptions that the try block can throw. Also, we can write as many catch blocks as required.
Example
In the following example, we have a division operation where the denominator is zero. We enclose this code in a try block.
To catch the exception thrown by this code, we write a catch block following try block. Since the code can throw DivisionByZeroError, we catch the same in the catch block.
PHP Program
<?php
try {
$a = 10;
$b = 0;
$c = $a / $b;
echo $c;
} catch (DivisionByZeroError $e) {
echo $e->getMessage();
}
?>
Output
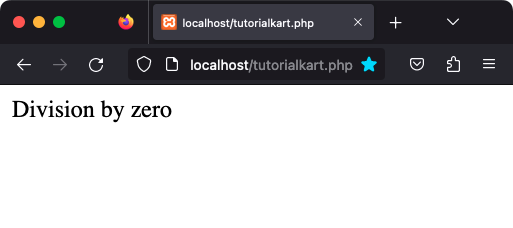
When there was an exception, respective catch block is found and that catch block is executed. The program execution is not terminated, but continues with the statements after try-catch.
If we did not have the try-catch block, then the expression $a / $b
would have thrown the DivisionByZeroError and terminated the program execution, as shown in the following program, since there is no provision for exception handling.
PHP Program
<?php
$a = 10;
$b = 0;
$c = $a / $b;
echo $c;
?>
Output – xampp/logs/php_error_log
[01-Mar-2023 06:04:47 Europe/Berlin] PHP Fatal error: Uncaught DivisionByZeroError: Division by zero in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php:4
Stack trace:
#0 {main}
thrown in /Applications/XAMPP/xamppfiles/htdocs/tutorialkart.php on line 4
The text written to the php_error_log clearly states that the DivisionByZeroError is uncaught with details to the part of the code that raised the error.
More Tutorials on Try Catch
There are some more variations to the try-catch statement that we mentioned above. We shall discuss those in the following tutorials.
- PHP – Multiple catch blocks PHP Tutorial to write multiple catch blocks after a try block.
- PHP – Try Catch with Final block
- PHP – Catch multiple exceptions in one catch block PHP Tutorial to write a catch block that can handle more than one exception.
Conclusion
In this PHP Tutorial, we learned how to use a try-catch statement to handle exceptions thrown by potential code.