In this tutorial, you shall learn about Inheritance in PHP, an Object Oriented Programming concept, how it can be implemented in PHP programs, with examples.
Inheritance
Inheritance is a way in which a new class (child class or subclass) can be created to inherit all the properties and methods of the existing class (parent class or superclass).
To implement inheritance in PHP, we can use extends keyword.
Example
In the following example, we create two classes: ParentClass and ChildClass. As the name suggests, ChildClass extends/inherits ParentClass, and therefore extends the properties and methods of the ParentClass.
PHP Program
<?php
class ParentClass {
// properties and methods
}
class ChildClass extends ParentClass {
// properties and methods
}
?>
Now, let us define some properties and methods for the ParentClass. Then we create a new object $child1
of type ChildClass, and access the property $x
and function sayHello()
of the ParentClass using ChildClass object $child1
.
PHP Program
<?php
class ParentClass {
public $x = 10;
public function sayHello() {
print_r("Hello from Parent class.\n");
}
}
class ChildClass extends ParentClass {
}
$child1 = new ChildClass();
$child1->sayHello();
print_r($child1->x);
?>
Output
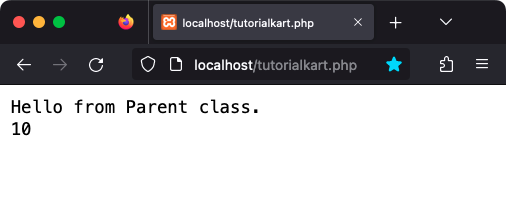
The above program demonstrates how a child class can access the properties and methods of its parent class.
We can override the properties and methods of a parent class in child class. For that you need to redefine the properties or methods that you would like to override in the child class.
In the following program, we define the property $x
and method sayHello()
in the child class, and thus override the property and method of the parent class.
PHP Program
<?php
class ParentClass {
public $x = 10;
public function sayHello() {
print_r("Hello from Parent class.\n");
}
}
class ChildClass extends ParentClass {
public $x = 20;
public function sayHello() {
print_r("Hello from Child class.\n");
}
}
$child1 = new ChildClass();
$child1->sayHello();
print_r($child1->x);
?>
Output
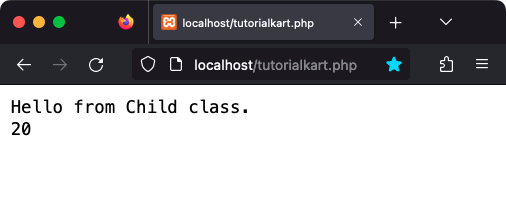
In the above program, we learned how to override properties and methods of a parent class in child class.
Conclusion
In this PHP Tutorial, we learned what Inheritance of Object Oriented Programming is, how to implement Inheritance in PHP, how to extend a class, what is a child class is, and what a parent class is, how to access the properties and methods of parent class from child class object, how to override the properties and methods of parent class in child class.