In this tutorial, you shall learn about PHP sizeof() function which can get the size of an array, with syntax and examples.
PHP sizeof() Function
The PHP sizeof() function returns number of elements in given array. We can also call it as size of array. You can also count recursively or not, if the array is nested, using sizeof() function.
The sizeof() function is an alias of the count() function.
Syntax of sizeof()
The syntax of sizeof() function is
sizeof( array, mode )
where
Parameter | Description |
---|---|
array | [mandatory] Specifies the array |
mode | [optional] The default value is COUNT_NORMAL. If you pass COUNT_RECURSIVE for mode, then the counting happens recursively for the nested arrays as well. |
Function Return Value
sizeof() returns the number of elements in given array.
Examples
1. Find the size of an Array
In this example, we will take an array with elements, and find the number of elements in it using sizeof() function.
PHP Program
<?php
$array1 = array("apple","banana");
$result = sizeof($array1);
echo $result;
?>
Output
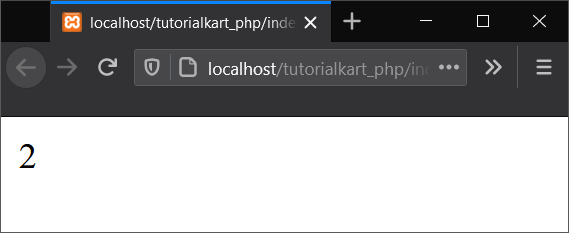
2. Recursively find number of elements in Nested Array
In this example, we will take an nested arrays, and find the number of elements in it recursively using sizeof() function.
PHP Program
<?php
$array1 = array("apple","banana",
array(12, 14, 17),
array("a", "b", "c", "d"),
);
$result = sizeof($array1, COUNT_RECURSIVE);
echo $result;
?>
Output
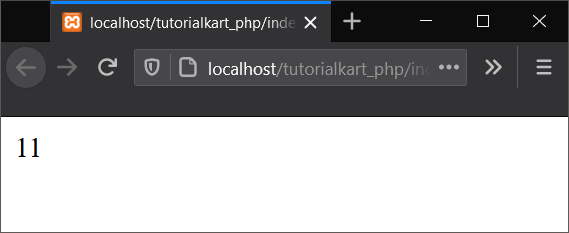
There are 4 elements in the outer array, 3 elements in array(12, 14, 17), and 4 elements in array(“a”, “b”, “c”, “d”). Total elements = 4+3+4 = 11.
Conclusion
In this PHP Tutorial, we learned how to find the size of a given array, using PHP sizeof() array function.