In this tutorial, you shall learn how to check if given array is empty in PHP using count() function, with the help of example programs.
PHP – Check if array is empty
To check if an array is empty or not in PHP, we can use count() function. count() function can be used to find the length of an array. If the length of an array is zero, then the array is empty.
The syntax of the condition to check if an array arr
is empty is
count($arr) == 0
Examples
1. Check if array is empty
In this example, we will take an array arr
with no elements in it, and programmatically check if this array arr
is empty or not using count()
function.
PHP Program
<?php
$arr = [];
if ( count($arr) == 0 ) {
echo "Array is empty.";
} else {
echo "Array is not empty.";
}
?>
Output
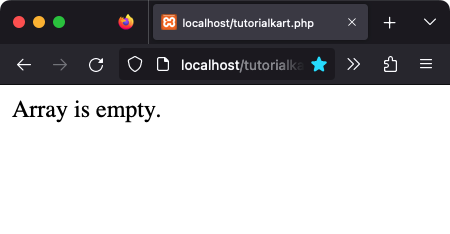
2. Take a non-empty array and check if array is empty
In this example, we will take an array arr
with some elements in it, and programmatically check if this array arr
is empty or not using count()
function.
PHP Program
<?php
$arr = ["apple", "banana"];
if ( count($arr) == 0 ) {
echo "Array is empty.";
} else {
echo "Array is not empty.";
}
?>
Output
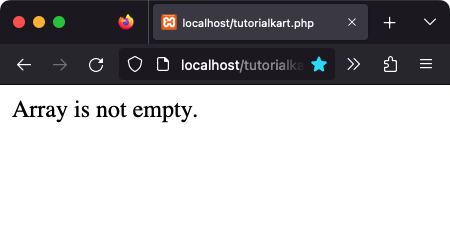
Conclusion
In this PHP Tutorial, we learned how to check if an array is empty or not using count()
function.