In this tutorial, you shall learn how to get current timestamp in PHP using time() or microtime() functions, with example programs.
PHP – Get Current Timestamp
To get the current timestamp in PHP, we can use date/time functions.
In this tutorial, we will go through the following date/time functions to get the current timestamp.
- time() – returns the current time as a Unix timestamp
- microtime() – returns the current Unix timestamp with microseconds
Get Current Timestamp
time()
function returns the current time in the number of seconds since Unix Epoch (1st Jan, 1970, 00:00:00 GMT).
PHP Program
<?php $timestamp = time(); echo $timestamp; ?>
Output
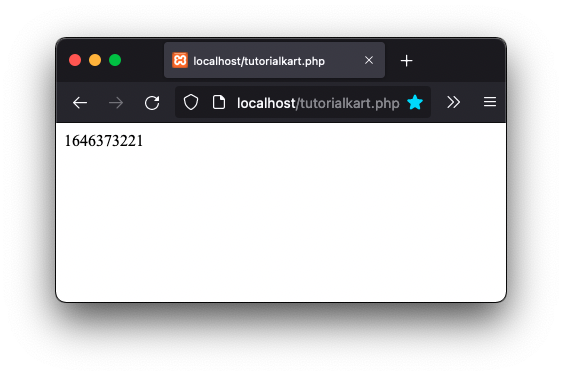
We can format this into required format using date() function.
PHP Program
<?php $timestamp = time(); $formatted = date('y-m-d h:i:s T', $timestamp); echo $formatted; ?>
Output
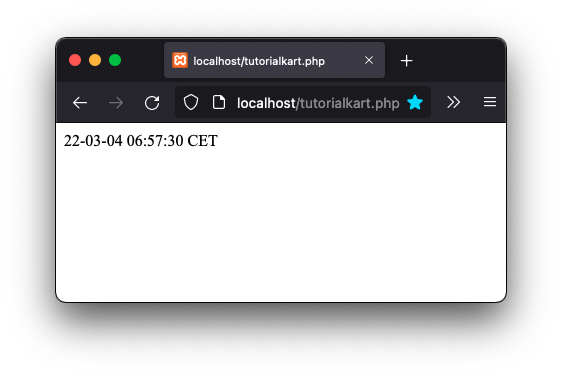
We can also use a predefined format like DATE_ATOM with date() function.
PHP Program
<?php $timestamp = time(); $formatted = date(DATE_ATOM, $timestamp); echo $formatted; ?>
Output
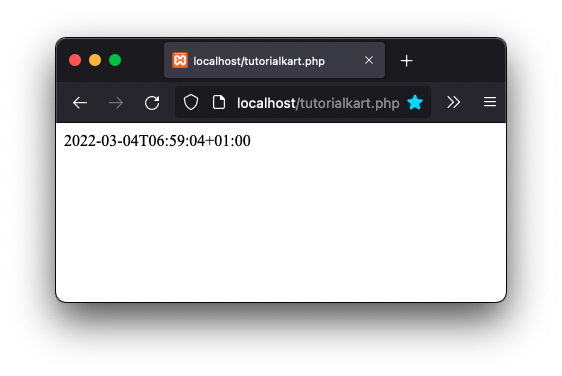
Get Current Timestamp in Microseconds
microtime()
function returns microseconds followed by the current time in the number of seconds since Unix Epoch (1st Jan, 1970, 00:00:00 GMT). We can extract the microseconds and seconds separately, and format accordingly.
PHP Program
<?php $timestamp = microtime(); list($u, $s) = explode(" ", $timestamp); $formatted = date('y-m-d h:i:s', (int) $s); $u = str_replace("0.", ".", $u); $formatted = $formatted . $u; echo 'microtime() : ', $timestamp; echo '<br>'; echo 'Unix Timestamp : ', $s; echo '<br>'; echo 'Microseconds : ', $u; echo '<br>'; echo 'Formatted : ', $formatted; ?>
Output
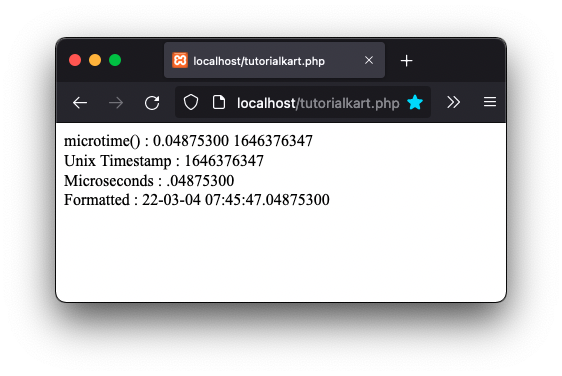
Conclusion
In this PHP Tutorial, we learned how to get the current timestamp in PHP, using time()
and microtime()
functions.