In this tutorial, you shall learn how to find all the substrings that match a given pattern in a string in PHP using preg_match_all() function, with the help of example programs.
PHP – Find all the substrings that match a pattern
To find all the substrings that match a given pattern in a string in PHP, we can use preg_match_all() function.
Call the preg_match_all() function and pass the pattern, input string, and variable to store the matchings, as arguments.
Syntax
The syntax to find all the substrings that match a given pattern $pattern
in the input string $str
is
preg_match_all($pattern, $str, $matches)
All the matchings and sub-matchings that match the given pattern are stored in the variable $matches
.
Examples
1. Find all the alphabetical words in the string
Let us define that a word contains only alphabetical characters, uppercase or lowercase, and contains one or more characters.
In this example, we take an input string in str
and take a pattern that matches with one or more alphabetical characters in $pattern
. We find the index of last occurrence of the search string search
in the string str
.
PHP Program
<?php
$str = "Apple 5236, Banana 41, Cherry 100";
$pattern = "/([a-z]+)/i";
if(preg_match_all($pattern, $str, $matches)) {
$words = $matches[0];
print_r($words);
}
?>
Output
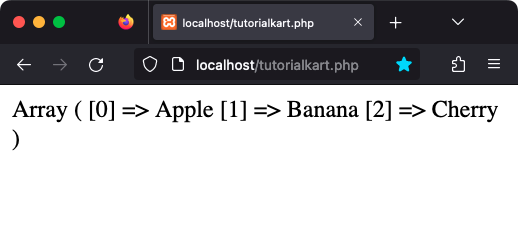
Reference to tutorials related to program
2. Find all the numeric values in the string
Let us define that a numeric value contains only numbers [0-9], and can be surrounded by non-numeric characters.
In this example, we find all the numeric values in the string $str
using preg_match_all() function.
PHP Program
<?php
$str = "Apple 5236, Banana 41, Cherry 100";
$pattern = "/([0-9]+)/";
if(preg_match_all($pattern, $str, $matches)) {
$words = $matches[0];
print_r($words);
}
?>
Output
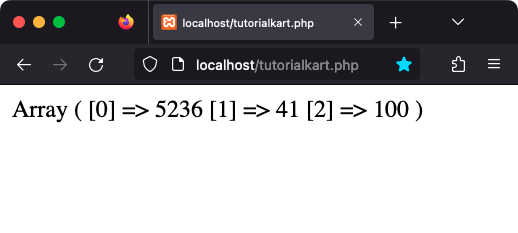
Reference to tutorials related to program
Conclusion
In this PHP Tutorial, we learned how to find all the substrings in a string that match a given pattern using preg_match_all() function, with the help of examples.