In this PHP tutorial, you will learn how to use NOT operator in If-statement condition, and some example scenarios.
PHP If NOT
PHP If statement with NOT operator is used to execute if-block if the condition is false. NOT operator with condition inverts the result of condition.
If statement condition with NOT operator
The following is a typical If-statement with NOT logical operator used in the condition.
if ( ! condition ) {
//if-block statement(s)
}
where
- condition can be simple conditional expression or compound conditional expression.
!
is the logical NOT operator in PHP. It takes only one operand: condition.
Since we are using OR operator to inverse the result of condition, PHP executes if-block if condition is false.
Examples
1. Check if value in a is not 2
In this example, we will write an if statement with compound condition. The compound condition contains two simple conditions and these are joined by OR logical operator.
PHP Program
<?php
$a = 3;
if ( ! ( $a == 2 ) ) {
echo "a is not 2.";
}
?>
Output
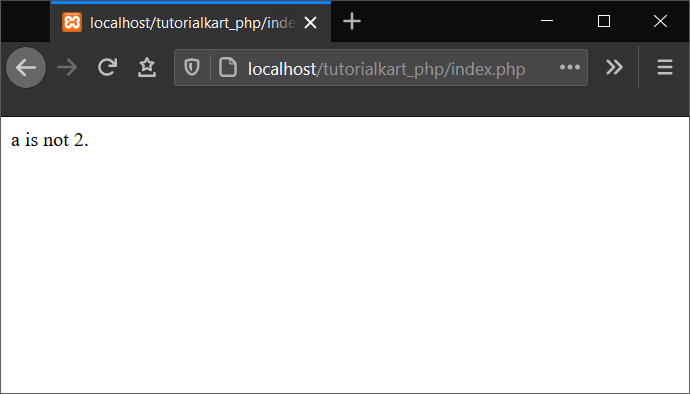
2. Check if the string does not contain “apple”
In this example, we will write an if statement with compound condition. The compound condition contains two simple conditions and these are joined by OR logical operator.
PHP Program
<?php
$name = "banana";
if ( $name !== "apple" ) {
echo "$name is not apple.";
}
?>
Output
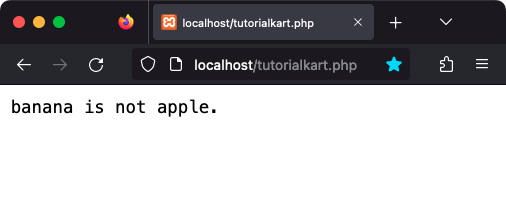
Conclusion
In this PHP Tutorial, we learned how to write PHP If statement with AND logical operator.