In this tutorial, you shall learn how to solve PHP error “Notice: Undefined offset: 0” when working with arrays.
PHP Array – Notice: Undefined offset: 0
You will get PHP message “Notice: Undefined offset” when you try to access an element with an offset or index that is not present in the PHP Array. We shall go through the scenarios where this could happen, and discuss how to solve it.
1. Accessing Array with Default Offset
For example, consider the following PHP program.
<?php $arr = array(25, 87, 63); echo $arr[4]; ?>
We have defined an array with some numbers. As we have not specified any offset to the elements, the default offset will start from 0 and would be 0,1 and 2 for the three elements respectively.
The offset range is from 0 to 2, but we are trying to access element of $arr at offset 4. This could echo a Notice message.
When you run the above program, you will get the following message displayed in the browser.
“Notice: Undefined offset: 4 “
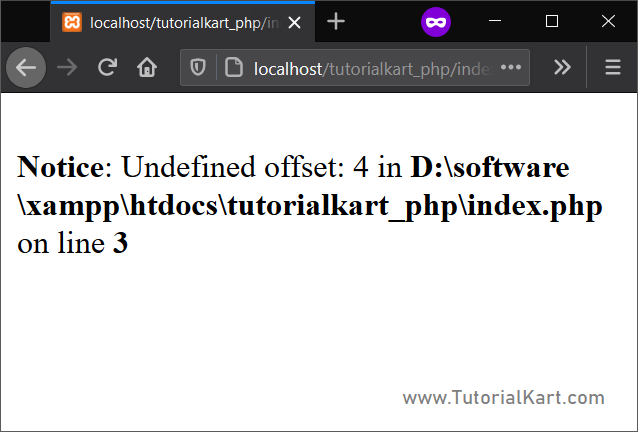
As specified by the message, we are trying to access an element at offset 4. But the array we defined has only three elements with offsets 0,1 and 2 respectively.
Solution
We can only access elements with the offsets 0,1 or 2.
PHP Program
<?php $arr = array(25, 87, 63); echo $arr[0]; echo '<br>'; echo $arr[1]; echo '<br>'; echo $arr[2]; echo '<br>'; ?>
Program Output
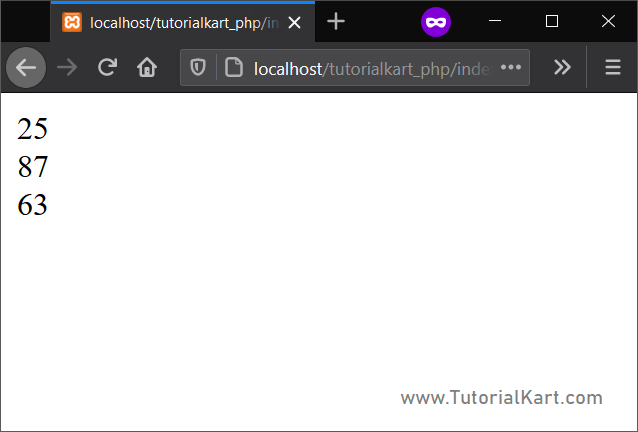
2. Accessing array with defined offset
You know that in PHP array, we can define the offset during array creation. For example, consider the following PHP program.
PHP Program
<?php $arr = array( 1 => 25, 87, 63 ); echo $arr[0]; ?>
We have defined an array with three numbers and specified the offset of first element to be 1. So, the next elements would get an offset of 2 and 3 respectively.
The offset range is from 1 to 3, but we are trying to access element of $arr at offset 0. This could echo a Notice message.
When you run the above program, you will get the following message displayed in the browser.
“Notice: Undefined offset: 0 “
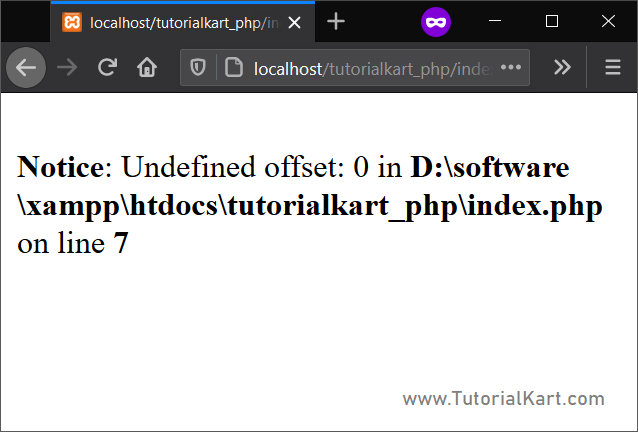
As specified by the message, we are trying to access an element at offset 0. But the array we defined has three elements with offsets 1, 2 and 3 respectively.
Solution
We can only access elements with the offsets 1, 2 or 3.
PHP Program
<?php $arr = array( 1 => 25, 87, 63 ); echo $arr[1]; echo '<br>'; echo $arr[2]; echo '<br>'; echo $arr[3]; echo '<br>'; ?>
Program Output
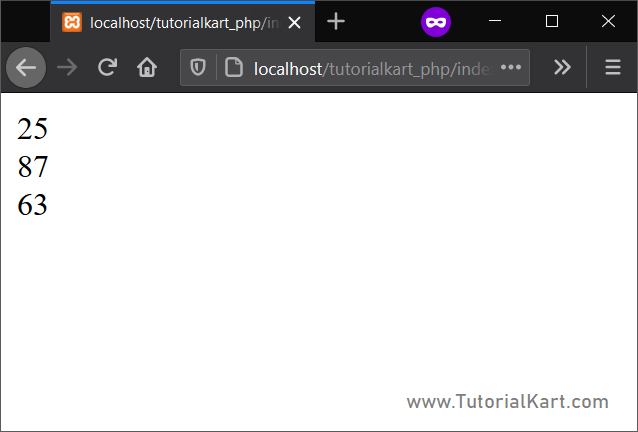
3. Accessing Array of key:value pairs
We can define a PHP array with key-value pairs. For example, consider the following PHP program.
PHP Program
<?php $arr = array( "a" => 25, "b" => 87, "c" => 63 ); echo $arr[0]; ?>
We have defined an array with three key-value pairs. So, we have to access the values of key-value pairs using key. But, if we try to access elements using index, just like we are trying to access $arr[0], we will get Notice message from the echo statement.
When you run the above program, you will get the following message displayed in the browser.
“Notice: Undefined offset: 0 “
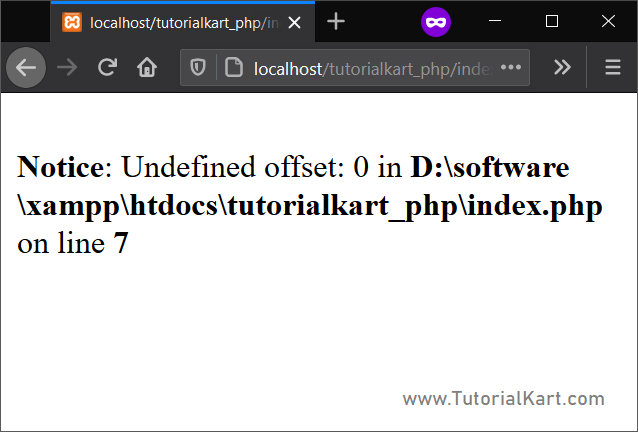
As specified by the message, we are trying to access an element at offset 0. But the array we defined has three key-value pairs with keys “a”, “b” and “c” respectively.
Solution
We can only access elements with the keys “a”, “b” or “c”.
PHP Program
<?php $arr = array( "a" => 25, "b" => 87, "c" => 63 ); echo $arr["a"]; echo "<br>"; echo $arr["b"]; echo "<br>"; echo $arr["c"]; echo "<br>"; ?>
Program Output
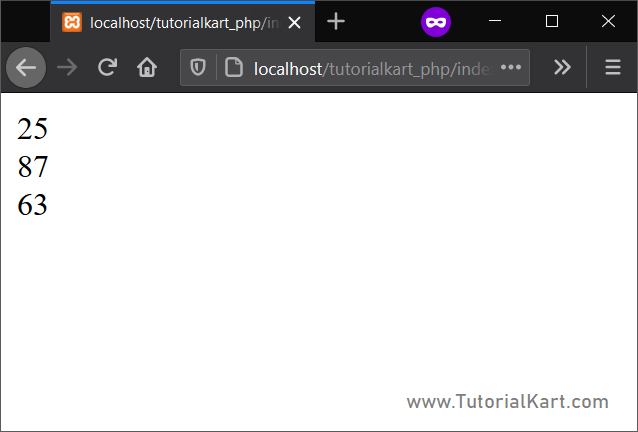
Conclusion
In this PHP Tutorial, we learned how to solve the “Notice: Undefined offset” when working with arrays.