In this tutorial, you shall learn about PHP array_diff_assoc() function which can compute the difference of an array against other arrays, with syntax and examples.
PHP array_diff_assoc() Function
PHP array_diff_assoc() function computes the difference of an array against other arrays. Both key/index and value are considered for comparison.
In this tutorial, we will learn the syntax of array_diff_assoc(), and how to use this function to find the difference of an array from other arrays, covering different scenarios based on array type and arguments.
Syntax of array_diff_assoc()
The syntax of PHP array_diff_assoc() function is
array_diff_assoc ( array $array1 , array $array2 [, array $... ] ) : array
where
Parameter | Description |
---|---|
array1 | [mandatory] Array of interest. Compare the items of this array against other arrays’. |
array2 | [mandatory] Array of reference. The items of this array are used to comparison against. |
Along with array2, you can provide as many number of arrays to compare against. But these additional arrays are optional.
Return Value
The array_diff_assoc() function returns an array whose key-value pairs are present in $array1 but not present in $array2 or other arrays(if provided).
Examples
1. Compute difference of Arrays: array1 – array2
In this example, we will take an associative array, array1, with key-value pairs, and compare it against another array, array2.
PHP Program
<?php
$array1 = array('a'=>'apple', 'b'=>'banana', 'c'=>'cherry');
$array2 = array('a'=>'apricot', 'b1'=>'banana', 'c'=>'cherry');
$result = array_diff_assoc($array1, $array2);
print_r($result);
?>
Output
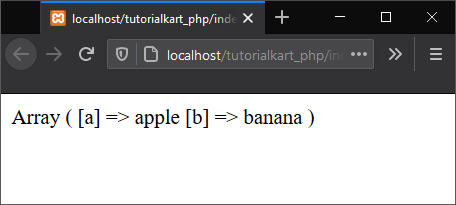
There are two observations that we can draw from this output. They are
'a'=>'apple'
and'a'=>'apricot'
differ in the value part.'b'=>'banana'
and'b1'=>'banana'
differ in the key part.
2. Compute difference of an Array against Multiple Arrays
In this example, we will take an array: array1 and find the difference of this array against three arrays.
PHP Program
<?php
$array1 = array('a'=>'apple', 'b'=>'banana', 'c'=>'cherry', 'd'=>'date');
$array2 = array('a'=>'apricot', 'b'=>'berry');
$array3 = array('c'=>'cherry', 'd'=>'dragon');
$array4 = array('e'=>'eggfruit', 'a'=>'apple');
$result = array_diff_assoc($array1, $array2, $array3, $array4);
print_r($result);
?>
Output
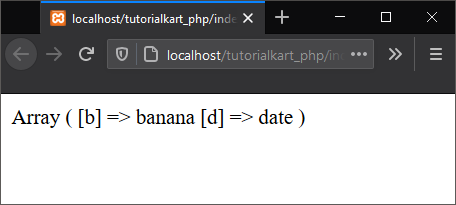
'a'=>'apple'
is present in array4, and 'c'=>'cherry'
is present in array3. Rest of the key-value pairs of array1 are not present in any of the arrays [array2, array3, array4]. So, those key-value pairs of array1, that are not present in other arrays are returned in the resulting array.
3. Compute difference of Indexed Arrays
In this example, we will take indexed arrays and find their difference. array_diff_assoc() will consider key or index to compute difference. In our previous examples, we have take associative arrays, so key is considered in those programs. But here, we are taking indexed arrays. So, index will be considered while computing difference.
For a better understanding, we have printed out the input arrays as well with index representation.
PHP Program
<?php
$array1 = array(5, 7, 1, 9, 3);
$array2 = array(5, 7, 3, 9, 3);
$result = array_diff_assoc($array1, $array2);
print_r($array1);
print_r($array2);
print_r($result);
?>
Output
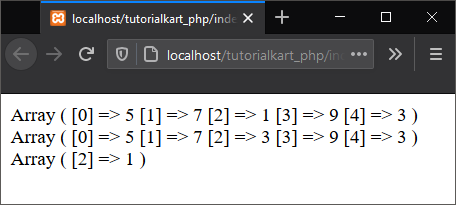
Conclusion
In this PHP Tutorial, we learned how to compute the difference of arrays considering key/index along with value, using PHP Array array_diff_assoc() function.