In this tutorial, you shall learn how to check if a variable is set in PHP using isset() function, with the help of example programs.
PHP – Check if variable is set
To check if a variable is set in PHP, pass the variable as argument to isset() function. The function returns a Boolean value of true if the variable is set, or else it returns false.
Syntax
The syntax to use isset() function to check if variable $x
is a set or not is
isset($x)
Example
In the following program, we take an integer value in variable $x
, and programmatically check if this variable is set or not.
PHP Program
<?php
$x = 48;
if ( isset($x) ) {
echo "variable x is set.";
} else {
echo "variable x is not set.";
}
?>
Output
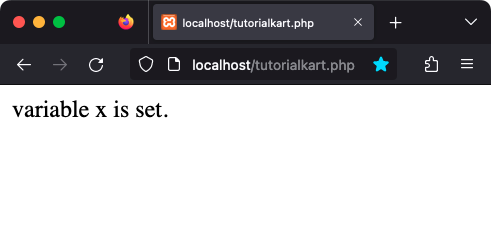
Now, let us check if variable $y
is set or not. In the following program, we have not taken any variable named $y
. Therefore, else block must execute.
PHP Program
<?php
if ( isset($y) ) {
echo "variable y is set.";
} else {
echo "variable y is not set.";
}
?>
Output
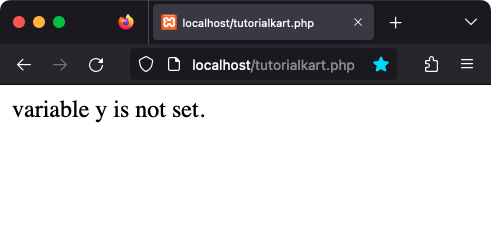
Conclusion
In this PHP Tutorial, we learned how to check if a variable is set or not, using isset() function.