In this tutorial, you shall learn how to output a string in PHP using echo() function, with syntax and example programs.
PHP echo
In PHP, echo
takes one or more expressions that evaluate to string, and outputs them. If any of the expression is not a string, then it is implicitly converted to string.
Syntax
The syntax of echo is
//echo single value echo($expression); //echo multiple values echo($expression_1, $expression_2, $expression_3); //echo without parenthesis echo $expression; echo $expression_1, $expression_2, $expression_3;
where
Parameter | Description |
---|---|
$expression | An expression to be output. Typically a string value. If not a string, then it is converted to a string to print to output. |
echo
does not return a value.
Usually, echo
without parenthesis is used.
Examples
1. Echo a string “Hello World” to output
In the following example, we print a string "Hello World"
to output using echo
.
PHP Program
<?php echo 'Hello World'; ?>
Output
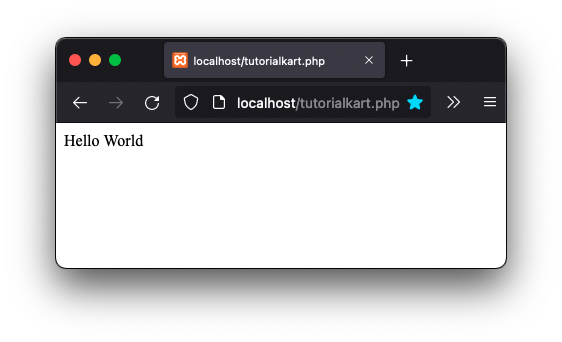
2. Echo multiple strings to output
In the following example, we print multiple strings using echo
.
PHP Program
<?php echo 'apple', 'banana', 'cherry'; ?>
Output
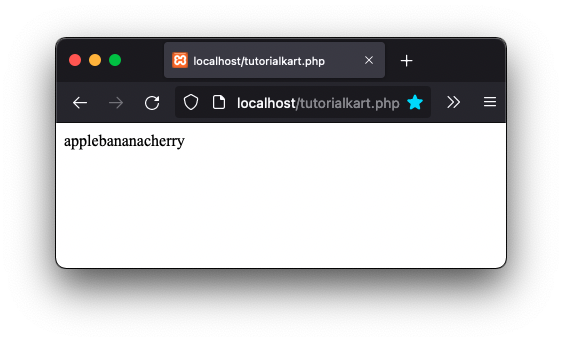
3. Echo variables to output
In the following example, we take values in variables, and print them using echo
.
PHP Program
<?php $name1 = 'apple'; $name2 = 'banana'; $name3 = 'cherry'; echo $name1, '-', $name2, '-', $name3; ?>
Output
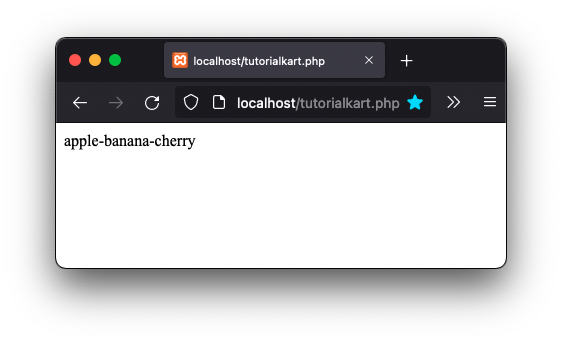
We have given variables, and string values to echo
simultaneously to print to output.
Conclusion
In this PHP Tutorial, we learned how to print one or more expressions to output, using echo
, with examples.