In this tutorial, you shall learn about Arithmetic Multiplication Operator in PHP, its syntax, and how to use this operator in PHP programs, with examples.
PHP Multiplication
PHP Arithmetic Multiplication Operator takes two numbers as operands and returns their product.
Symbol
*
symbol is used for Multiplication Operator.
Syntax
The syntax for Multiplication Operator is
operand_1 * operand_2
The operands could be of any numeric datatype, integer or float.
If the two operands are of different datatypes, implicit datatype promotion takes place and value of lower datatype is promoted to higher datatype.
Examples
1. Multiplication of Integers
In the following example, we take integer values in $x
and $y
, and find their product $x * $y
using Arithmetic Multiplication Operator.
PHP Program
<?php $x = 5; $y = 4; $output = $x * $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x * y = $output"; ?>
Output
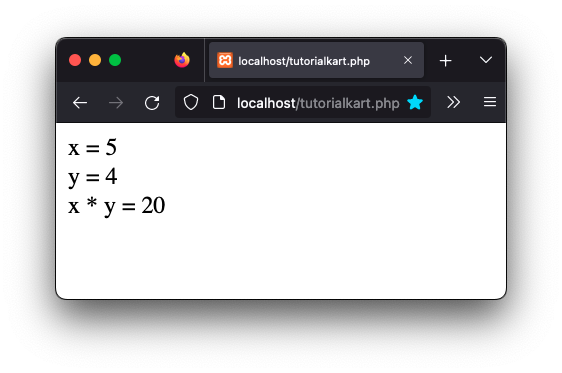
2. Multiplication of Float Values
In the following example, we take float values in $x
and $y
, and find their product $x * $y
.
PHP Program
<?php $x = 5.1; $y = 4.2; $output = $x * $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x * y = $output"; ?>
Output
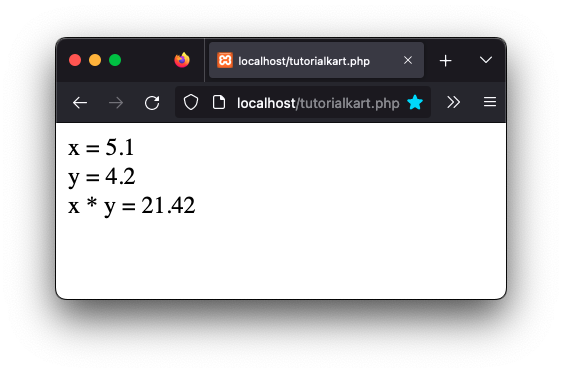
3. Multiplication of Integer and Float Values
In the following example, we take integer value in $x
and floating point value in $y
, and add their product $x * $y
.
Since float is higher datatype among integer and float, the output is float.
PHP Program
<?php $x = 5; $y = 4.21; $output = $x * $y; echo "x = $x" . "<br>"; echo "y = $y" . "<br>"; echo "x * y = $output"; ?>
Output
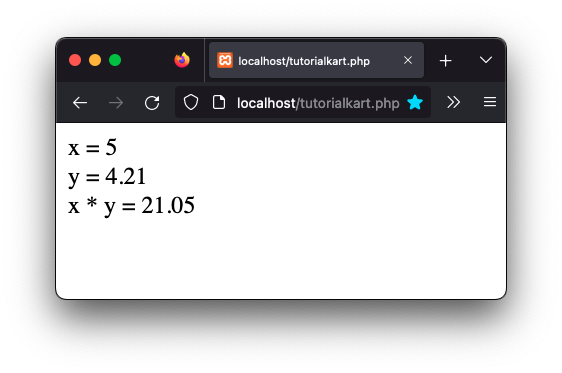
Conclusion
In this PHP Tutorial, we learned how to use Arithmetic Multiplication Operator to find the product of two numbers.