In this PHP tutorial, you shall learn about Indexed Arrays, how to created indexed arrays, access elements of indexed arrays, and modify elements of indexed arrays, with example programs.
PHP – Indexed Arrays
Indexed Arrays are arrays in which the elements are ordered based on index. The index can be used to access or modify the elements of array.
Create Indexed Array
To create an indexed array in PHP, use array() function with the comma separated elements passed as argument to the function.
The syntax to create indexed array using array() function is
$myarray = array(element_1, element_2, element_3)
In the above code snippet, array() function returns an indexed array.
Let us write a PHP program, where we shall create an indexed array using array() function.
PHP Program
<?php
$myArray = ["apple", "banana", "orange", "mango", "guava"];
?>
Access elements of Indexed Array
In the following example, we will access elements of the array using index.
PHP Program
<?php
//indexed array
$myArray = ["apple", "banana", "orange", "mango", "guava"];
//access element using index
$myElement = $myArray[2];
echo $myElement;
?>
Output
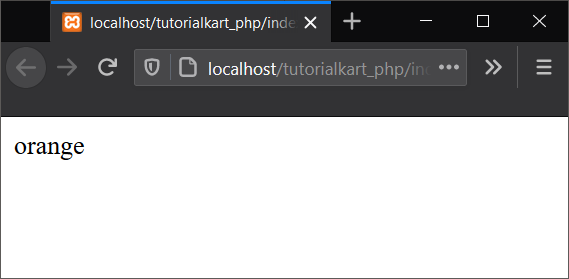
Modify elements of Indexed Array
To modify the elements of array using index, assign a new value to the array variable followed by the index in square brackets.
In the following example, we will initialize an indexed array, and update the element at index 2, i.e., third element.
PHP Program
<?php
//indexed array
$myArray = ["apple", "banana", "orange", "mango", "guava"];
//modify element using index
$myArray[2] = "papaya";
//print array elements
foreach ($myArray as $element) {
echo $element;
echo "<br>";
}
?>
Output
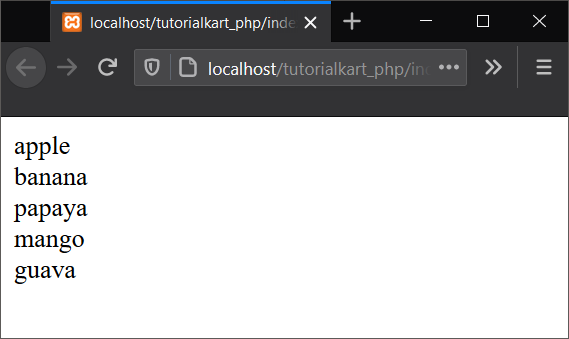
Conclusion
In this PHP Tutorial, we learned about indexed arrays in PHP, how to use index to access of modify elements of Indexed Arrays, with the help of example PHP programs.