In this PHP tutorial, you shall learn how to check if given string contains one or more numbers using ctype_digit() function, with example programs.
PHP – Check if string contains numbers
To check if string contains numbers, check if there is any character that is a digit. Iterate over the characters of the string, and for each character, use ctype_digit() function to check if the character is a digit or not. When you found a digit, you can break the loop and confirm that there is a number in the string.
ctype_digit() function returns true if the string passed to it has digits for all of its characters, else it returns false. In this tutorial, we will pass each character of the string, to ctype_digit() function, as a string.
Examples
1. Check if given string has number(s)
In this example, we will take a string, “apple123”, write a for loop to iterate over individual characters of the string, and for each character check if it is a digit, using ctype_digit() function.
PHP Program
<?php
$string = "apple123";
$isThereNumber = false;
for ($i = 0; $i < strlen($string); $i++) {
if ( ctype_digit($string[$i]) ) {
$isThereNumber = true;
break;
}
}
if ( $isThereNumber ) {
echo "\"{$string}\" has number(s).";
} else {
echo "\"{$string}\" does not have number(s).";
}
?>
Output
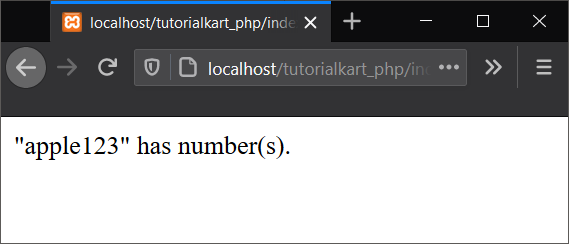
Conclusion
In this PHP Tutorial, we learned how to check if a string contains number(s) or not, using ctype_digit() built-in PHP function.