In this PHP tutorial, you shall learn how to split a given string into words using str_split() function, with example programs.
PHP – Split String into Words
To split a string into words in PHP, use explode() function with space as delimiter. The explode() function returns an array containing words as elements of the array.
The following is a step by step process to split given string into words.
- Take a string with words. We will split this string into words.
- Define the delimiter. Usually, space would be delimiter between words in a string. But, you can assign the required value for this variable, that works as delimiter for words in a given string.
- Call explode() function, with the delimiter and string passed as arguments.
- explode() returns an array containing words. Save it to a variable.
Examples
1. Split string “Apple is healthy.” into words
In this example, we will take a string containing words. We will explode this string with space as delimiter, collect the words into a variable, and print them using echo.
PHP Program
<?php
$str = "Apple is healthy.";
$delimiter = ' ';
$words = explode($delimiter, $str);
foreach ($words as $word) {
echo $word;
echo "<br>";
}
?>
Output
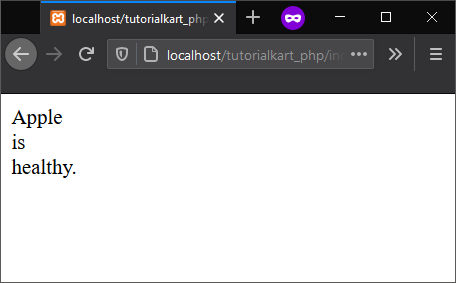
You may clean the words from punctuation marks. Also, you may convert the string to a lower case, so that the words would be uniform with respect to case.
Conclusion
In this PHP Tutorial, we learned how to split a string into words, using explode() function.