In this tutorial, you shall learn how to create an array in PHP using array() constructor, how to create one dimensional and two dimensional arrays, with examples.
PHP Create Array
To create an array in PHP, use array() function.
We will discuss through multiple scenarios on how to create an array in PHP using array(), based on the indexing it generates for its elements.
Create Array using array() function
The syntax of array() function is
array ([ mixed $... ] )
where for mixed
parameter, you can provide any of these values
- comma separated key => value pairs
- comma separated values
array() function returns a PHP array created using the arguments passed to it.
In the following example, we will create an array containing a mix of integers and strings.
PHP Program
<?php $arr = array( 85, "apple", "banana" ); ?>
In the following example, we will create an array with key => value pairs.
PHP Program
<?php $arr = array( "a" => 58, "b" => 99, "c" => 41 ); ?>
Create Two Dimensional Arrays
A two dimensional array is an array in which one or more values inside the array is another array.
PHP Program
<?php $arr = array( "fruits" => array("apple", "banana", "cherry"), "numbers" => array(54, 99, 31), "names" => array("Jack", "Arya", "Arjun") ); ?>
Create PHP array with Automatic Array Index for elements
Automatic Array indexing is a mechanism in which index is generated for the array elements based on the index that we may or may not provide to the elements in the array. Based on the type of index we provide, there are many scenarios we can go through Automatic Array Indexing. We shall go through each of them with examples.
1. Index is omitted
In this scenario, we create an array of element with no index specified. In such case, PHP automatically creates an integer index for the elements. The integer index starts at 0, incrementing by one for the next elements.
In the following example, we created an array with elements, but not provided any index. So, the index of first element is 0, index of second element is 1, index of third element is 2, and so on.
PHP Program
<?php $arr = array( "apple", "banana", "cherry" ); foreach ($arr as $key => $value) { echo $key . ' - ' . $value . '<br>'; } ?>
Program Output
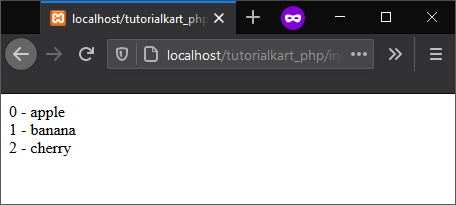
2. Index provided to first element
In this scenario, index is provided to first element in the array. In such case, the given index is taken as starting index and the next elements will be assigned with next integers.
Following is an example program where we created an array with elements, and gave an index of 5 to first element. So, the index of first element would be 5, the next element’s is 6, and the next element’s index is 7.
PHP Program
<?php $arr = array( 5 => "apple", "banana", "cherry" ); foreach ($arr as $key => $value) { echo $key . ' - ' . $value . '<br>'; } ?>
Program Output
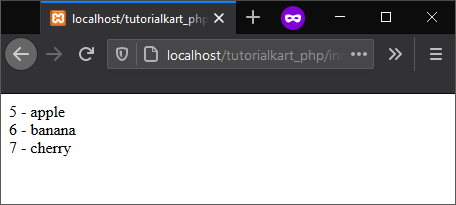
3. Index provided to an element somewhere in the middle of array
In this scenario, index is provided to an element in the array whose position is not the first. In such case, this index acts as starting index from this element’s position and the next elements will get next integers as their index. But for the elements prior to that element, the first case “index is omitted” will be applied.
PHP Program
<?php $arr = array( "apple", "banana", "cherry", 6 => "mango", "orange", "grape" ); foreach ($arr as $key => $value) { echo $key . ' - ' . $value . '<br>'; } ?>
Program Output
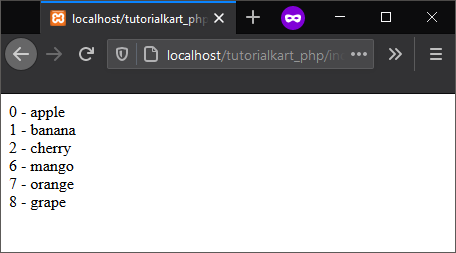
4. Same Index provided to multiple elements in array
In this scenario, same index is given to multiple elements in the array. In such case, the later key => value pair is considered to update the previous element with the same index.
PHP Program
<?php $arr = array( "apple", "banana", 6 => "cherry", "fig", 6 => "mango", "orange", "grape" ); foreach ($arr as $key => $value) { echo $key . ' - ' . $value . '<br>'; } ?>
Program Output
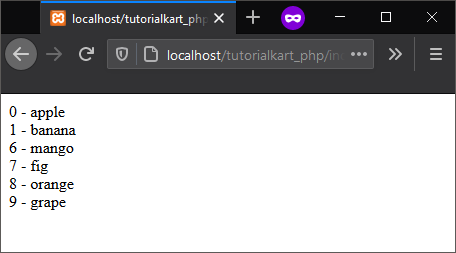
Let us break down this scenario and understand how indexing is given to the elements.
For the first two elements, no index is provided. So, their index start with zero. Hence 0 for “apple”, and 1 for “mango”.
We have given an index of 6 for “cherry”.
There is no index for “fig”, so increment previous index by one and assign it to “fig”.
Now, “mango” has an index of 6, but we already have an element with index 6. So, the existing element with index 6 will be updated with the new value “mango”. As a result, “mango” replaces “cherry”.
“orange” and “grape” do not have index, so they get an incremented index from the previous element. And the index would be 8 and 9 respectively.
Concluding this scenario, when different elements got same index, the later will update the prior one.
5. Non integer Index
In this scenario, we provide index of type string to some of the elements. The elements which do not have get an integer index based on the first scenario “Index is omitted”.
PHP Program
<?php $arr = array( "apple", "banana", "xc" => "cherry", "fig", "xm" => "mango", "orange", "grape" ); foreach ($arr as $key => $value) { echo $key . ' - ' . $value . '<br>'; } ?>
Program Output
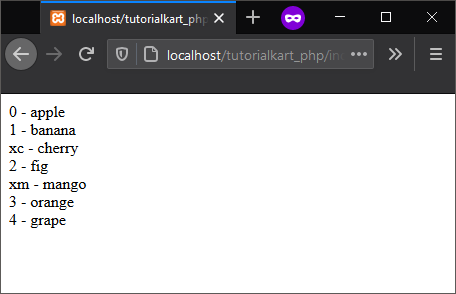
Conclusion
In this PHP Tutorial, we learned how to create a PHP array using array() function.