In this tutorial, you shall learn how to filter elements in a given array based on a condition in PHP using array_filter() function, with the help of example programs.
PHP – Filter elements in array based on a condition
To filter elements in given array based on a condition in PHP, we can use array_filter() function.
The syntax to filter elements in an array arr
using array_filter()
function is
array_filter($arr, callback_function, flag);
where
- callback_function is a function that receives each value of the input array as argument.
If the callback function returns true for given value (item), then that item will be present in the returned array by array_filter()
function, else if the callback function returns false for a value, then that value will not be present in the returned array.
Note: The key or index of the elements from the original array remain unchanged in filtered array.
Examples
1. Filter even numbers in an array
In this example, we take an array with numbers arr
, and filter only even numbers in this array using array_filter()
function.
PHP Program
<?php $arr = [4, 5, 2, 1, 3, 6, 8, 10]; $filtered_array = array_filter($arr, function ($item) { return $item % 2 == 0; }); foreach ($filtered_array as $value) { echo $value . ", "; } ?>
Output
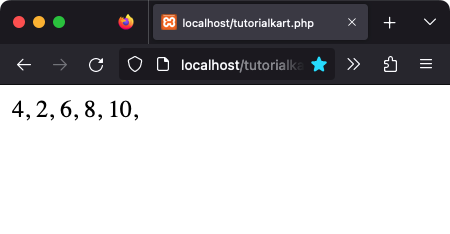
2. Filter strings in an array whose length is greater than 4
In this example, we take an array with strings, and filter only those strings whose length is greater than 4 using array_filter()
function.
PHP Program
<?php $array = ["car", "aeroplane", "motorbike", "jeep"]; $length = 4; $filtered_array = array_filter($array, function ($item) use ($length) { return strlen( $item ) > $length; }); foreach ($filtered_array as $value) { echo $value . ", "; } ?>
Output
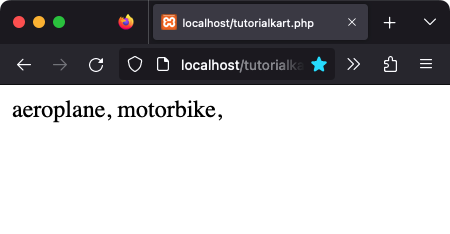
Conclusion
In this PHP Tutorial, we learned how to filter elements in an array, using PHP array_filter() function.