In this PHP tutorial, you shall learn about For loop statement, its syntax, execution flow, nested For loop, etc., with example programs.
For Loop in PHP
The for loop executes a block a statements in a loop multiple times.
Of course, you can mention the initial values with which a for loop can start, mention a condition based on which for loop decides when to continue with or stop the loop, and mention an update where you can update the loop control variable(s).
Syntax of For Loop
The syntax of for loop is
for (initialization; condition; update) {
statement(s)
}
Execution Flow of For Loop
initialization is executed only once, during the start of for loop. During each loop execution, condition is verified. If the condition returns true, then the statement(s) inside for loop are executed. After executing the statement(s), update section is executed. Then it continues with the condition evaluation. The loop is broken when the condition becomes false, or if the for loop is explicitly broken by a statement (we shall discuss about this scenario later).
Examples
1. A simple example of a For Loop to print numbers from 1 to 5
Let us write a simple PHP program with a for loop, where we print numbers from 1 to 5. Here printing a number is the process that we need to execute in a loop. Initialization, condition, and update sections can be derived from the range of number we would like to print.
As we need to start printing from 1, we shall initialize the control variable with 1. And, we have to print numbers in increments of 1, so, we will increment the control variable in the update section. We have to stop the execution after printing 5. So, we shall define condition such that the loop executes when the control variable is less than 5.
We have to convert these statements into code.
PHP Program
<?php
for ( $x = 1; $x <= 5; $x++ ) {
echo $x;
echo "<br>";
}
?>
Output
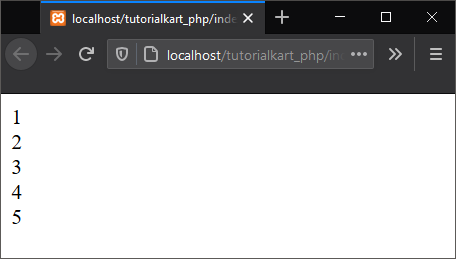
In the above example,
$x
is the control variable.$x = 1
is the initialization.$x <= 5
is the condition.$x++
is the update to control variable.
2. For Loop to iterate over elements of an array
Using for loop, we can traverse through the elements of array. Index of array sets the initialization, condition and update, just like in our previous example.
In the following example, we shall create an array, and loop through its elements using for loop.
PHP Program
<?php
$arr = ["apple", "banana", "orange"];
for ( $x = 0; $x < sizeof($arr); $x++ ) {
echo $arr[$x];
echo "<br>";
}
?>
Output
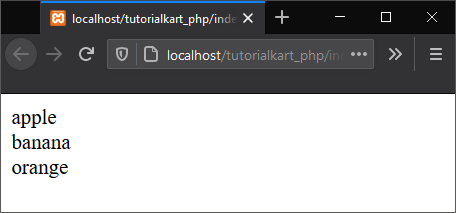
Nested For Loop
We can write for loop inside a for loop. This is called nesting.
In the following program, we will write a nested for loop, and print a triangular star pattern.
PHP Program
<?php
for ( $x = 0; $x < 5; $x++ ) {
for ( $y = 0; $y <= $x; $y++ ) {
echo "* ";
}
echo "<br>";
}
?>
Output
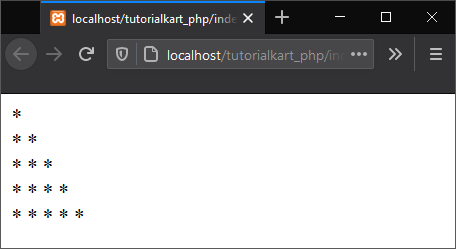
Conclusion
In this PHP Tutorial, we learned what a for loop is, its syntax, and how to use a for loop with example programs.