In this tutorial, you shall learn how to convert a PHP array into a JSON string using json_encode() function, with syntax and example programs.
PHP – Convert Array into JSON String
To convert an associative array into a JSON String in PHP, call json_encode()
function and pass the associative array as argument. This function can take other optional parameters also that effect the conversion to JSON String.
Syntax
The syntax of json_decode()
function is
json_encode(value, flags, depth)
where
Parameter | Description |
---|---|
value | [Required] The value which has to be encoded into a JSON String. |
flags | [Optional] Bitmask consisting of JSON_FORCE_OBJECT , JSON_HEX_QUOT , JSON_HEX_TAG , JSON_HEX_AMP , JSON_HEX_APOS , JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_NUMERIC_CHECK , JSON_PARTIAL_OUTPUT_ON_ERROR , JSON_PRESERVE_ZERO_FRACTION , JSON_PRETTY_PRINT , JSON_UNESCAPED_LINE_TERMINATORS , JSON_UNESCAPED_SLASHES , JSON_UNESCAPED_UNICODE , JSON_THROW_ON_ERROR . |
depth | [Optional] Integer. Specifies the maximum depth. Default value = 512. |
json_encode()
returns a string, or false
if the encoding is not successful.
Examples
1. Convert given Associative array into JSON string
In this example, we take an associative array and convert it into a JSON string using json_encode()
function with the default optional parameters. We shall display the string returned by json_encode()
in the output.
PHP Program
<?php
$arr = array("apple" => 25, "banana" => 41, "cherry" => 36);
$output = json_encode($arr);
echo $output;
?>
Output
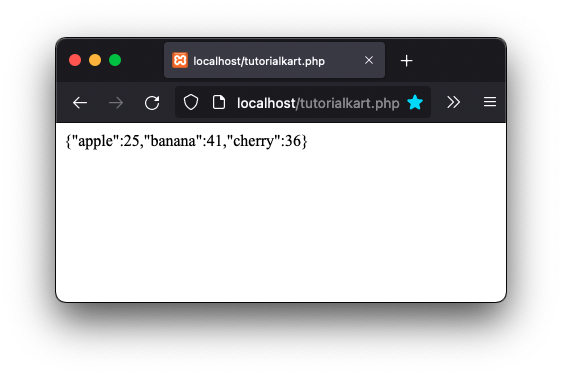
2. Convert nested Associative array into JSON string
In this example, we take a nested associative array, where the value for a key in the outer array is another array, and convert it into a JSON string using json_encode()
function.
PHP Program
<?php
$arr = array(
"a" => 25,
"b" => 41,
"c" => array(
"d" => 12,
"e" => 58
),
);
$output = json_encode($arr);
echo $output;
?>
Output
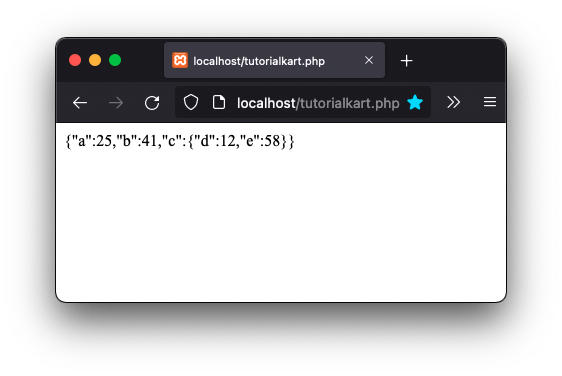
Conclusion
In this PHP Tutorial, we learned how to convert an array into a JSON string, using json_encode()
function.