In this tutorial, you shall learn about PHP array_count_values() function which can count the occurrences of all values in an array, with syntax and examples.
PHP array_count_values() Function
PHP array_count_values() function counts the occurrences of all values in an array and returns an associative array formed by the unique value of input array as keys, and the number of their occurrences in the array as values.
In this tutorial, we will learn the syntax of array_count_values(), and how to use array_count_values() to count the occurrences of values in the array, covering different scenarios based on array type and arguments.
Syntax of array_count_values()
The syntax of PHP array_count_values() function is
array_count_values ( array $input ) : array
where
Parameter | Description |
---|---|
input | Occurrences of values in this input array are counted. |
Return Value
The array_count_values() function returns an array formed with unique values as keys, and their number of occurrences as values.
Warnings
array_count_values() expects elements of the array have to be either string or integer. So, if an element is neither string nor integer, the function throws a warning.
Examples
1. Count the occurrences of values in array $input
In this example, we will take a array and count the occurrences of values in the array.
PHP Program
<?php
$input = array(41, 'a', 41, 'a', 'b');
$result = array_count_values($input);
print_r($result)
?>
Output
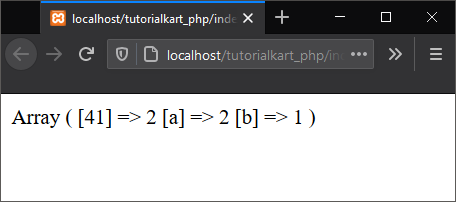
The value 41
has occurred two times, a
has occurred two times and b
has occurred one time in the input array.
There are two observations that we can draw from this output. They are
- The value of the input array has become keys in the resulting array.
- The number of occurrences of each value in the input array, has become the values in the resulting array.
3. Count values in given Associative Array
In this example, we will take an associative array with key-value pairs, and call array_count_values(). The keys of associative array are ignored and only the values are considered for counting.
PHP Program
<?php
$input = array(
'key1'=>41,
'key2'=>'a',
'key3'=>41,
'key4'=>'a',
'key5'=>'b'
);
$result = array_count_values($input);
print_r($result)
?>
Output
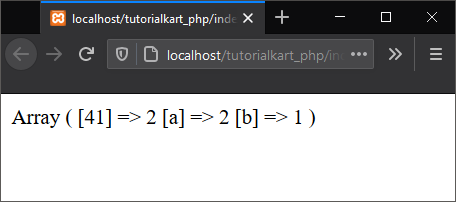
3. Warning: array_count_values(): Can only count STRING and INTEGER values
array_count_values() can count only STRING and INTEGER values. Warning shall be raised for values, in the array, of any other datatypes.
In the following example, we have an array with one float value, and the rest are strings and integers. call array_count_values() function will throw a warning for the first value, and continues with counting for the string and integer values.
PHP Program
<?php
$input = array(41.235, 'a', 41, 'a', 'b');
$result = array_count_values($input);
print_r($result)
?>
Output
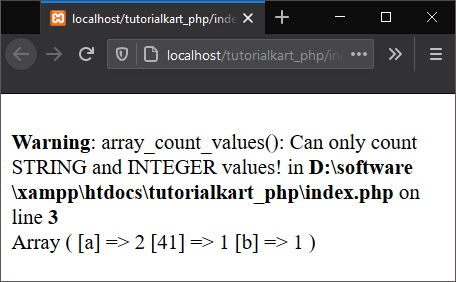
Conclusion
In this PHP Tutorial, we learned how to count occurrences of values in a given array, using PHP Array array_count_values() function.