In this tutorial, you shall learn how to find the index of last occurrence of a search string in a string in PHP using strrpos() function, with the help of example programs.
PHP – Find index of last occurrence in a string
To find the index of last occurrence in a string in PHP, call strrpos()
function and pass the string and search string as arguments.
If the search string is present in the string, then the strrpos()
function returns an integer value representing the index of the search string in the string, else the function returns a boolean value of false.
Syntax
The syntax to find the index of last occurrence of $search
string in the $str
string is
strrpos($str, $search)
Examples
1. Find index of last occurrence in string (String contains search string)
In this example, we take string values in str
and search
. We find the index of last occurrence of the search string search
in the string str
.
PHP Program
<?php $str = 'apple banana apple cherry'; $search = 'apple'; $index = strrpos($str, $search); echo "Last Index : " . $index; ?>
Output
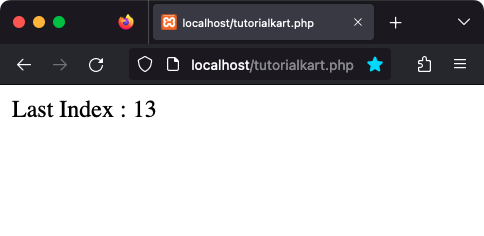
Reference tutorials for this program
2. Find index of last occurrence in string (String does not contain search string)
In this example, we take a string in str
and search string search
, such that the string str
does not contain search
. So, in this case, when we try to find the index of last occurrence of search string using strrpos(), the function returns false.
To check if the function strrpos() returned false or an integer, use PHP If statement.
PHP Program
<?php $str = 'apple banana apple cherry'; $search = 'mango'; $index = strrpos($str, $search); if ($index !== false) { echo "Last Index : " . $index; } else { echo "String does not contain search string."; } ?>
Output
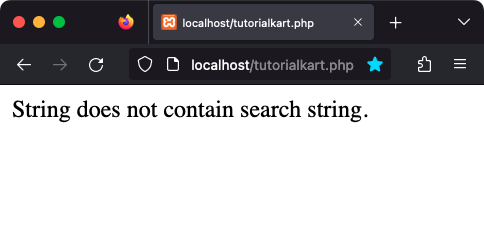
Reference tutorials for this program
Conclusion
In this PHP Tutorial, we learned how to find the index of last occurrence in a string, using strrpos() function, with the help of examples.