In this PHP tutorial, you shall learn how to check if given string contains only alphabet characters using preg_match() function, with example programs.
PHP – Check if string contains only English Alphabets
To check if a string contains only English language alphabets in PHP, call preg_match()
function and pass pattern and given string as arguments. The pattern must match one or more uppercase or lowercase alphabets from starting to end of the string.
Syntax
The syntax to check if string str
contains only english alphabets is
preg_match('/^[A-Za-z]+$/', $str)
The above expression returns a boolean value of true
if the string $str
contains only alphabets, or false
otherwise.
Examples
1. Positive Scenario – String contains only English alphabets
In this example, we take a string in str
such that it contains only uppercase and/or lowercase English alphabets. We shall check programmatically if str
contains only English alphabets.
For the given value of string, preg_match()
returns true
and if-block executes.
PHP Program
<?php $str = 'appleBanana'; $pattern = '/^[A-Za-z]+$/'; if ( preg_match($pattern, $str) ) { echo 'string contains only alphabets'; } else { echo 'string does not contain only alphabets'; } ?>
Output
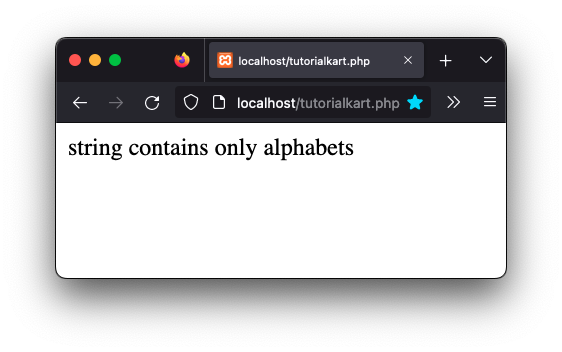
2. Negative Scenario – String contains not only English alphabets
In this example, we take a string in str
such that it contains characters other than uppercase and/or lowercase English alphabets. We shall check programmatically if str
contains only English alphabets.
For the given value of string, preg_match()
returns false
and else-block executes.
PHP Program
<?php $str = 'apple 123'; $pattern = '/^[A-Za-z]+$/'; if ( preg_match($pattern, $str) ) { echo 'string contains only alphabets'; } else { echo 'string does not contain only alphabets'; } ?>
Output
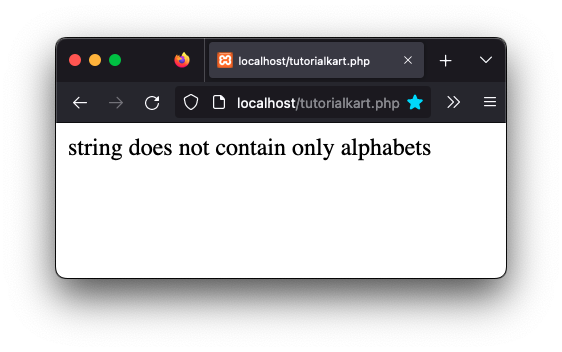
Conclusion
In this PHP Tutorial, we learned how to check if given string contains only alphabets, using preg_match()
function, with the help of examples.