In this tutorial, you shall learn how to write nested foreach statements in PHP, with the help of example programs.
PHP – Nested foreach
Nested foreach is a statement in which we have a foreach as statement inside a foreach.
Nested foreach can used to iterate over values of multidimensional arrays in PHP.
Examples (2)
ADVERTISEMENT
1. Iterate over 2D Indexed Array using nested foreach
Let us take a two-dimensional array, and use nested foreach to traverse the elements of the array.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); foreach ($array2D as $array1D) { foreach ($array1D as $element) { echo $element; echo " "; } echo "<br>"; } ?>
Output
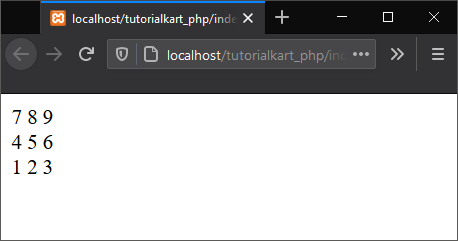
2. Iterate over an Associative Array where the values are Arrays using foreach
In the following program, we take a two dimensional array and iterate over the elements in inner-arrays using nested foreach statement.
PHP Program
<?php $arr = array( "a" => array( 41, 96, 65 ), "b" => array( 88, 44, 22 ), "c" => array( 12, 23, 34 ) ); foreach ($arr as $key => $value) { echo $key . '<br>'; foreach ($value as $element) { echo $element . ' '; } echo '<br>'; } ?>
Output
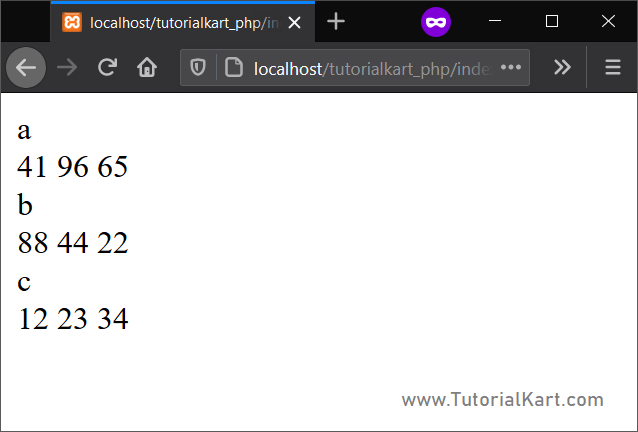
Conclusion
In this PHP Tutorial, we learned what a nested foreach statement is, and how to use this statement to iterate over a multidimensional array.